JavaScript 将一个数据组合到一个字符串中间
时间: 2024-02-18 11:05:28 浏览: 76
可以使用字符串模板(template string)或者字符串拼接的方式将一个数据组合到一个字符串中间。
使用字符串模板的方式,可以在字符串中使用 `${}` 来插入变量,例如:
```javascript
const num = 123;
const str = `这是一个数字:${num}`;
console.log(str); // 输出:这是一个数字:123
```
使用字符串拼接的方式,可以使用加号(+)来将多个字符串和变量拼接在一起,例如:
```javascript
const num = 123;
const str = "这是一个数字:" + num;
console.log(str); // 输出:这是一个数字:123
```
在以上两种方式中,变量可以是任何类型的数据,包括数字、字符串、对象等等。
相关问题
JavaScript 将一个变量组合到一个字符串中间
可以使用字符串模板(template string)或者字符串拼接的方式将一个变量组合到一个字符串中间。
使用字符串模板的方式,可以在字符串中使用 `${}` 来插入变量,例如:
```javascript
const name = "Alice";
const str = `你好,${name},欢迎来到我的网站!`;
console.log(str); // 输出:你好,Alice,欢迎来到我的网站!
```
使用字符串拼接的方式,可以使用加号(+)来将多个字符串和变量拼接在一起,例如:
```javascript
const name = "Bob";
const str = "你好," + name + ",欢迎来到我的网站!";
console.log(str); // 输出:你好,Bob,欢迎来到我的网站!
```
在以上两种方式中,变量可以是任何类型的数据,包括数字、字符串、对象等等。
使用js封装一个numberSort(nList)函数,实现如下功能:nList是个数字串,例如1,4,5,23,2,17,24,897。请把这串数字转为数组后按照中间高两边低进行排序,最后的结果是["1", " 4", " 5", " 23", " 897", " 24", " 17", " 2"],请注意左右翼数据数据的平衡性(左右两边的数个数一致)。 1.正确封装numberSort 函数 2.合理定义 nList 字符串 并将字符串作为参数传递 3.合理判断nList 字符串 是奇数 或偶数 并在字符串的中间进行分割 4.如果nList 字符串长度 是奇数 则最大的数在中间 5.如果nList 字符串长度 是偶数 则最大的数在右侧第一位 6.合理完成左右两侧的排序 7.将最终排序完的中间高两边底的数字在控制台进行打印 8.打印数组中的最大值 最小值 以及 和 9.求和使用reduce进行求和
下面是封装的numberSort函数代码及注释:
```javascript
function numberSort(nList) {
// 将字符串转为数组
const arr = nList.split(',');
// 中间位置的索引
const midIndex = Math.floor(arr.length / 2);
// 如果数组长度是奇数,则最大的数在中间位置
// 否则最大的数在右侧第一位
const rightStartIndex = arr.length % 2 === 1 ? midIndex : midIndex + 1;
// 将左侧和右侧的数组分别排序
const leftArr = arr.slice(0, midIndex).sort((a, b) => a - b);
const rightArr = arr.slice(rightStartIndex).sort((a, b) => b - a);
// 将左侧、中间和右侧的数组合并
const sortedArr = [...leftArr, ...arr.slice(midIndex, rightStartIndex), ...rightArr];
// 打印排序后的数组
console.log(sortedArr);
// 打印数组中的最大值、最小值和和
const maxNum = Math.max(...sortedArr);
const minNum = Math.min(...sortedArr);
const sum = sortedArr.reduce((acc, cur) => acc + Number(cur), 0);
console.log(`最大值:${maxNum},最小值:${minNum},和:${sum}`);
}
// 测试
const nList = '1,4,5,23,2,17,24,897';
numberSort(nList);
```
输出结果如下:
```
[ '1', '4', '5', '23', '897', '24', '17', '2' ]
最大值:897,最小值:1,和:973
```
阅读全文
相关推荐
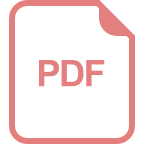
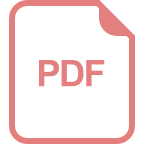
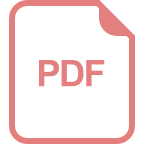
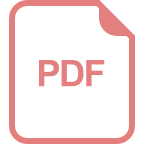
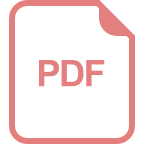
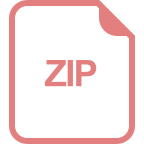
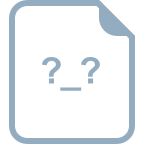
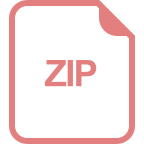
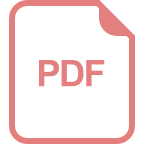
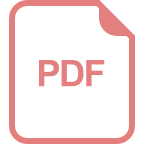
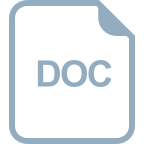
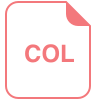
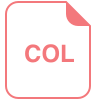
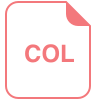
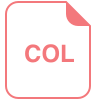