毫秒的时间戳换算时分秒毫秒,C语言实现
时间: 2024-12-18 20:23:24 浏览: 7
在C语言中,将毫秒时间戳转换成小时、分钟、秒和毫秒的形式,可以按照以下步骤操作:
```c
#include <stdio.h>
#include <time.h>
// 将毫秒转换为时间结构体 tm
struct tm time_from_milliseconds(long milliseconds) {
struct tm* t = localtime(&milliseconds);
// 时间戳包含年、月、日等信息,这里只关心小时、分钟、秒和毫秒
t->tm_year = -1900; // 从 1970 年开始计数,减去 1900
t->tm_mon = 0; // 月份从 0 开始
t->tm_mday = 1; // 日
t->tm_hour = t->tm_sec / 60;
t->tm_min = (t->tm_sec % 60) / 60;
t->tm_sec = (t->tm_sec % 60) % 60;
return *t;
}
// 输出时间格式
void print_time(struct tm* t) {
printf("%02d:%02d:%02d.%03d", t->tm_hour, t->tm_min, t->tm_sec, t->tm_usec);
}
int main() {
long milliseconds = your_timestamp_in_ms; // 替换成实际的毫秒值
// 转换并打印
struct tm time_struct = time_from_milliseconds(milliseconds);
print_time(&time_struct);
return 0;
}
```
在这个例子中,`your_timestamp_in_ms` 应该替换为你要转换的实际毫秒时间戳。注意这个代码片段假设输入的是自 Unix 纪元(1970年1月1日 00:00:00 UTC)以来的毫秒数。
阅读全文
相关推荐
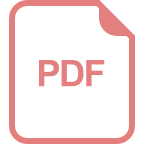
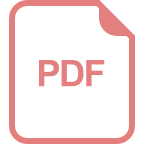
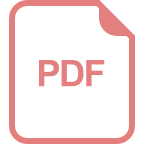
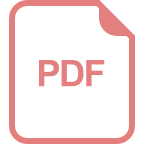










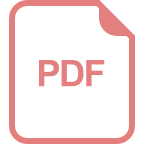

