java代码发送websocket请求
时间: 2023-08-31 14:05:23 浏览: 44
以下是Java代码发送WebSocket请求的示例:
```java
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Scanner;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.TimeUnit;
import org.java_websocket.client.WebSocketClient;
import org.java_websocket.handshake.ServerHandshake;
public class WebSocketExample {
public static void main(String[] args) throws URISyntaxException, InterruptedException {
// Replace this with the actual WebSocket URL
String webSocketUrl = "ws://example.com/websocket";
// Create a WebSocket client instance
WebSocketClient client = new WebSocketClient(new URI(webSocketUrl)) {
// The onOpen method is called when the WebSocket connection is established
@Override
public void onOpen(ServerHandshake handshakedata) {
System.out.println("WebSocket connection established");
}
// The onMessage method is called when a message is received from the WebSocket server
@Override
public void onMessage(String message) {
System.out.println("Received message: " + message);
}
// The onClose method is called when the WebSocket connection is closed
@Override
public void onClose(int code, String reason, boolean remote) {
System.out.println("WebSocket connection closed");
}
// The onError method is called when an error occurs on the WebSocket connection
@Override
public void onError(Exception ex) {
System.out.println("WebSocket error: " + ex.getMessage());
}
};
// Connect to the WebSocket server
client.connect();
// Wait for the WebSocket connection to be established
CountDownLatch latch = new CountDownLatch(1);
latch.await(5, TimeUnit.SECONDS);
// Send a message to the WebSocket server
Scanner scanner = new Scanner(System.in);
System.out.print("Enter message to send: ");
String message = scanner.nextLine();
client.send(message);
// Wait for the WebSocket connection to be closed
latch.await();
// Close the WebSocket connection
client.close();
}
}
```
在这个示例中,我们使用了 `org.java_websocket.client.WebSocketClient` 类来创建一个 WebSocket 客户端实例。我们覆盖了 `onOpen`、`onMessage`、`onClose` 和 `onError` 方法来处理 WebSocket 连接的各种事件。我们调用 `connect` 方法来连接到 WebSocket 服务器,然后使用 `CountDownLatch` 等待连接成功。我们使用 `Scanner` 类从控制台读取用户输入的消息,并使用 `send` 方法将消息发送到 WebSocket 服务器。最后,我们等待 `CountDownLatch` 以等待 WebSocket 连接关闭,并调用 `close` 方法来关闭 WebSocket 连接。
相关推荐
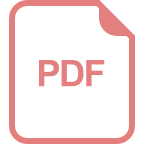
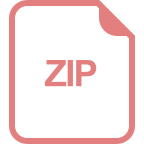
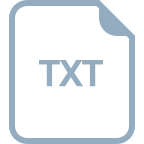












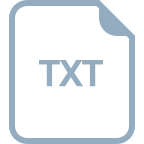
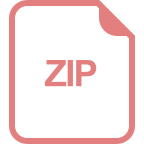
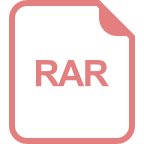