linux中如何用c语言写一个动态配置文件
时间: 2024-02-16 21:03:34 浏览: 96
在Linux中,可以使用C语言编写一个动态配置文件,主要步骤如下:
1. 定义配置文件的格式:可以采用INI格式或者JSON格式等。例如,可以定义一个INI格式的配置文件,包含若干个节和每个节下的键值对,如下所示:
```
[section1]
key1=value1
key2=value2
[section2]
key3=value3
key4=value4
```
2. 使用C语言中的文件操作函数,如fopen、fread、fwrite、fclose等打开、读取、写入和关闭文件。
3. 解析配置文件:使用C语言中的字符串操作函数,如strtok、strncpy、atoi等解析配置文件中的每个节和键值对,并将其存储到内存中的数据结构中,如数组、链表或哈希表等。
4. 对配置文件进行增、删、改、查等操作:使用C语言中的各种算法和数据结构库,如排序、搜索、插入、删除等操作,对配置文件进行动态修改。
5. 将修改后的配置文件保存到磁盘:使用C语言中的文件操作函数,将修改后的配置文件写入到磁盘中,以便下次程序启动时加载。
总之,动态配置文件的实现需要结合文件操作、字符串操作和数据结构等多种技术手段,需要充分考虑程序的可靠性和性能。
相关问题
linux c语言实现创建一个配置文件并实现读写
要实现创建一个配置文件并实现读写,可以使用C语言中的文件操作函数。具体步骤如下:
1. 使用 fopen 函数打开一个文件,如果文件不存在则创建文件。
2. 使用 fprintf 函数向文件中写入内容,格式可以自定义,如键值对、JSON等。
3. 使用 fseek 函数将文件指针移动到文件开头。
4. 使用 fgets 函数逐行读取文件内容,可以使用字符串处理函数解析出键值对等信息。
5. 使用 fclose 函数关闭文件。
下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define CONFIG_FILE "config.txt"
#define MAX_LINE_LENGTH 1024
int main() {
// 创建配置文件并写入内容
FILE *fp = fopen(CONFIG_FILE, "w+");
if (fp == NULL) {
printf("Failed to create config file!\n");
exit(1);
}
fprintf(fp, "name=John\n");
fprintf(fp, "age=30\n");
fprintf(fp, "city=New York\n");
fseek(fp, 0, SEEK_SET);
// 读取配置文件并解析内容
char line[MAX_LINE_LENGTH];
while (fgets(line, MAX_LINE_LENGTH, fp) != NULL) {
char *key = strtok(line, "=");
char *value = strtok(NULL, "=");
if (key != NULL && value != NULL) {
printf("Key: %s, Value: %s", key, value);
}
}
fclose(fp);
return 0;
}
```
上述代码创建了一个名为 "config.txt" 的配置文件,并向文件中写入了三个键值对。然后,程序将文件指针移动到文件开头,并逐行读取文件内容,解析出键值对并打印出来。最后,关闭文件并退出程序。
Linux C语言 读写配置文件
在Linux C语言中,读写配置文件通常使用INI文件格式。INI文件格式是一种简单的文本文件格式,其中键值对以节(section)的形式组织,并使用方括号([])将节名括起来。
以下是一个示例INI文件:
```
[Section1]
key1=value1
key2=value2
[Section2]
key3=value3
key4=value4
```
使用C语言读取INI文件的步骤如下:
1. 打开INI文件并读入内容。
2. 解析INI文件内容,将键值对存储到内存中的数据结构中。
3. 使用存储的键值对执行相应的操作。
以下是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1024
#define MAX_SECTION_LEN 256
#define MAX_KEY_LEN 256
#define MAX_VALUE_LEN 256
typedef struct {
char section[MAX_SECTION_LEN];
char key[MAX_KEY_LEN];
char value[MAX_VALUE_LEN];
} config_item_t;
int parse_config_file(const char* filename, config_item_t** items, int* count) {
FILE* fp = fopen(filename, "r");
if (!fp) {
return -1;
}
char line[MAX_LINE_LEN];
config_item_t* item = NULL;
int item_count = 0;
while (fgets(line, MAX_LINE_LEN, fp)) {
// 去掉行末换行符
char* p = strchr(line, '\n');
if (p) *p = '\0';
// 去掉行首空格
p = line;
while (*p == ' ') ++p;
// 解析节
if (*p == '[') {
char* q = strchr(p, ']');
if (!q) {
fclose(fp);
return -1;
}
*q = '\0';
item = NULL;
continue;
}
// 解析键值对
if (item) {
char* q = strchr(p, '=');
if (!q) continue; // 忽略无效行
*q = '\0';
strcpy(item->key, p);
strcpy(item->value, q + 1);
++item;
} else {
// 新建配置项
item = (config_item_t*)realloc(item, (item_count + 1) * sizeof(config_item_t));
if (!item) {
fclose(fp);
return -1;
}
strcpy(item->section, p);
item->key[0] = '\0';
item->value[0] = '\0';
items[item_count++] = item;
}
}
fclose(fp);
*count = item_count;
return 0;
}
void free_config_items(config_item_t** items, int count) {
for (int i = 0; i < count; ++i) {
free(items[i]);
}
free(items);
}
int main() {
config_item_t* items[100];
int count = 0;
if (parse_config_file("test.ini", items, &count) == 0) {
for (int i = 0; i < count; ++i) {
printf("[%s]\n", items[i]->section);
printf("%s=%s\n", items[i]->key, items[i]->value);
}
}
free_config_items(items, count);
return 0;
}
```
该程序使用`parse_config_file`函数读取INI文件并解析内容,将每个节的键值对存储到一个`config_item_t`结构体中。最后,在`main`函数中遍历所有配置项并输出。
阅读全文
相关推荐
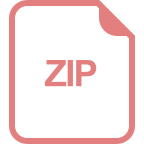
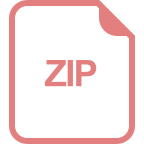
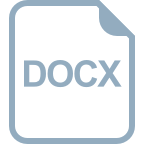













