@GetMapping("/{id}") public Result findOne(@PathVariable Integer id) { Integer userid = TokenUtils.getCurrentUser().getId(); Goods goods = goodsService.getById(id); List<Praise> praises = praiseService.list(); List<Collect> collects = collectService.list(); Praise praise = praiseService.getOne(new QueryWrapper<Praise>().eq("userid", userid).eq("goodsid", id)); Collect collect = collectService.getOne(new QueryWrapper<Collect>().eq("userid", userid).eq("goodsid", id)); goods.setHasPraise(praise != null); goods.setHasCollect(collect != null); goods.setPraiseCount(praises.stream().filter(p -> p.getGoodsid().equals(goods.getId())).count()); goods.setCollectCount(collects.stream().filter(c -> c.getGoodsid().equals(goods.getId())).count()); return Result.success(goods); }
时间: 2023-06-20 08:07:15 浏览: 194
这是一个基于Spring框架的Java后端代码,使用了@GetMapping注解来映射HTTP GET请求。其中,@PathVariable注解用于获取请求路径中的id参数,TokenUtils.getCurrentUser()用于获取当前登录用户的id,goodsService、praiseService、collectService都是该系统中的服务组件,用于管理商品、点赞、收藏等功能。在返回结果之前,代码会通过查询判断当前用户是否已经点赞、收藏过该商品,并计算出该商品的点赞数和收藏数,最终将结果封装在Result对象中返回给前端。
相关问题
@DeleteMapping("/{id}") public Result delete(@PathVariable Integer id) { ordersService.removeById(id); return Result.success(); } @PostMapping("/del/batch") public Result deleteBatch(@RequestBody List<Integer> ids) { ordersService.removeByIds(ids); return Result.success(); } @GetMapping public Result findAll() { return Result.success(ordersService.list()); } @GetMapping("/{id}") public Result findOne(@PathVariable Integer id) { return Result.success(ordersService.getById(id)); }
这是一个使用Spring Boot框架开发的RESTful API接口,包含了删除单个订单、批量删除订单、获取所有订单和获取单个订单的功能。其中,@DeleteMapping("/{id}")和@PathVariable注解实现了根据订单ID删除单个订单的功能;@PostMapping("/del/batch")和@RequestBody注解实现了批量删除订单的功能;@GetMapping注解实现了获取所有订单的功能;@GetMapping("/{id}")和@PathVariable注解实现了根据订单ID获取单个订单的功能。返回结果使用了自定义的Result类,其中的success()方法返回一个成功的响应结果。
@GetMapping("/{id}") public Result findOne(@PathVariable Integer id) { return Result.success(commentService.getById(id)); }
### 回答1:
这段代码是一个基于Spring框架的Java RESTful API的GET请求处理方法,用于获取一个id对应的评论。具体解释如下:
- @GetMapping("/{id}"):这是一个Spring注解,用于将HTTP的GET请求映射到该方法上,其中{id}是一个路径变量,表示该请求需要一个名为id的参数。
- public Result findOne(@PathVariable Integer id):这是该方法的定义,它接受一个类型为Integer的路径变量id作为参数,并返回一个Result对象,该对象包含了获取到的评论信息。
- return Result.success(commentService.getById(id)):这是该方法的具体实现,它调用了commentService对象的getById方法,获取到id对应的评论信息,并将评论信息封装到Result对象中返回给调用者。
总之,这段代码实现了一个GET请求处理方法,用于获取指定id的评论信息,并返回给调用者。
### 回答2:
@GetMapping("/{id}")是一个使用HTTP GET请求方法的注解,代表根据"id"来获取资源。在这个方法中,我们使用@PathVariable注解来获取URL路径中的"id"参数,并将其作为整数类型的参数传递给findOne方法。
在findOne方法内部,我们调用commentService的getById方法来根据传入的"id"参数进行查询操作,并将查询结果转换为Result对象返回。
假设我们有一个评论服务,其中包含评论的信息,每个评论都有一个唯一的整数类型的"id"。当我们使用HTTP GET请求访问"/{id}"路径时,我们可以通过调用findOne方法来获取指定"id"的评论信息。
例如,如果我们使用GET请求访问"/1"路径,findOne方法将会接收到"id"参数为1。然后,它将调用commentService的getById方法来查询数据库中"id"为1的评论信息,并将查询结果封装到一个Result对象中返回。作为结果,我们将在客户端收到一个被封装的评论信息。
这样,通过@GetMapping("/{id}")和findOne方法的配合使用,我们可以根据指定的"id"来获取相应的评论信息,并将其以Result对象的形式返回给客户端。
### 回答3:
@GetMapping("/{id}")是一个用于处理GET请求的注解,其中{id}是一个占位符,表示URL路径中的一个参数。当请求的URL路径匹配到这个注解时,会执行这个方法。
在这段代码中,public Result findOne(@PathVariable Integer id)是一个处理GET请求的方法,它接受一个名为id的Integer类型参数。@PathVariable注解用于将URL路径中的参数值赋值给方法参数id。
方法体中的commentService.getById(id)是通过id去数据库查询相应的评论信息。然后将查询结果通过Result.success()方法封装成一个成功的响应结果返回。
这段代码的作用是根据给定的id值,查询数据库中对应的评论信息,并将查询结果返回给调用者。如果查询成功,则返回成功的响应结果,否则返回失败的响应结果。
总之,这段代码用于处理GET请求,并根据给定的id查询相应的评论信息。返回结果经过封装后,以JSON格式返回给调用者。
阅读全文
相关推荐
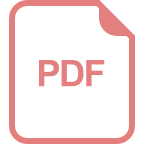
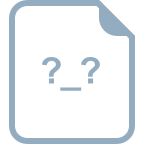
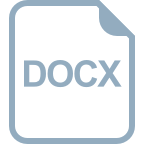





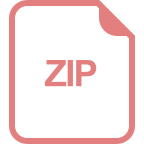
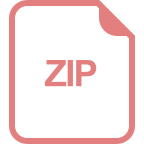
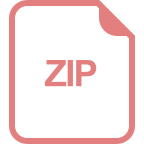