用python编写程序实现接收字符串并按字符出现频率的降序打印字母
时间: 2024-03-16 12:46:43 浏览: 93
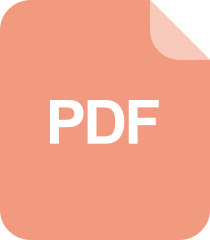
Python字符串对象实现原理详解
好的,这个问题可以用 Python 中的字典来解决。你可以使用 collections 模块中的 Counter 类来统计字符串中各个字符出现的次数,然后按照出现次数进行降序排列。
下面是一个示例代码:
```python
from collections import Counter
def print_char_by_freq(input_str):
# 统计字符出现次数
char_count = Counter(input_str)
# 按照出现次数降序排列
sorted_char_count = sorted(char_count.items(), key=lambda item: item[1], reverse=True)
# 打印结果
for char, count in sorted_char_count:
print(char, count)
# 测试代码
input_str = "hello world"
print_char_by_freq(input_str)
```
输出结果为:
```
l 3
o 2
h 1
e 1
1
w 1
r 1
d 1
```
代码说明:
1. 首先导入 collections 模块中的 Counter 类。
2. 定义一个函数 `print_char_by_freq`,接受一个字符串参数。
3. 使用 Counter 对象统计字符串中各个字符出现的次数,得到一个字典对象 `char_count`。
4. 使用 sorted 函数对字典对象中的键值对进行排序,按照值进行降序排列,得到一个列表对象 `sorted_char_count`。
5. 遍历排序后的列表对象,依次输出每个字符和对应的出现次数。
阅读全文
相关推荐
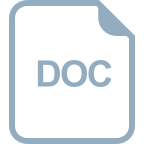
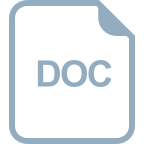















