java EasyExcel一段代码实现自定义色号 :蓝色,个性色1,淡色60%
时间: 2023-11-22 11:24:23 浏览: 37
使用EasyExcel可以轻松实现自定义颜色。以下是一个示例代码,其中包含自定义颜色的设置:
```java
import com.alibaba.excel.EasyExcel;
import com.alibaba.excel.write.style.row.SimpleRowHeightStyleStrategy;
import com.alibaba.excel.write.style.row.SimpleRowWidthStyleStrategy;
import com.alibaba.excel.write.style.row.SimpleVerticalCellStyleStrategy;
import org.apache.poi.ss.usermodel.*;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class ExcelColorExample {
public static void main(String[] args) throws IOException {
// 创建工作簿
Workbook workbook = WorkbookFactory.create(true);
// 创建工作表
Sheet sheet = workbook.createSheet("Sheet1");
// 创建自定义颜色
Color blue = new Color(0, 112, 192);
Color accent1 = new Color(5, 99, 193);
Color light60Percent = new Color(191, 191, 191);
// 将自定义颜色添加到调色板
Workbook wb = sheet.getWorkbook();
CreationHelper createHelper = wb.getCreationHelper();
IndexedColors.addCustomColor("blue", blue);
IndexedColors.addCustomColor("accent1", accent1);
IndexedColors.addCustomColor("light60Percent", light60Percent);
// 创建样式
CellStyle style = workbook.createCellStyle();
style.setFillForegroundColor(IndexedColors.BLUE.getIndex());
style.setFillPattern(FillPatternType.SOLID_FOREGROUND);
CellStyle style2 = workbook.createCellStyle();
style2.setFillForegroundColor(IndexedColors.ACCENT_1.getIndex());
style2.setFillPattern(FillPatternType.SOLID_FOREGROUND);
CellStyle style3 = workbook.createCellStyle();
style3.setFillForegroundColor(IndexedColors.LIGHT_TURQUOISE.getIndex());
style3.setFillBackgroundColor(IndexedColors.LIGHT_60_PERCENT.getIndex());
style3.setFillPattern(FillPatternType.SOLID_FOREGROUND);
// 创建行
Row row1 = sheet.createRow(0);
Row row2 = sheet.createRow(1);
Row row3 = sheet.createRow(2);
// 创建单元格
Cell cell1 = row1.createCell(0);
Cell cell2 = row2.createCell(0);
Cell cell3 = row3.createCell(0);
// 设置单元格样式
cell1.setCellStyle(style);
cell2.setCellStyle(style2);
cell3.setCellStyle(style3);
// 写入数据
cell1.setCellValue("蓝色");
cell2.setCellValue("个性色1");
cell3.setCellValue("淡色60%");
// 调整行高和列宽
sheet.setDefaultRowHeightInPoints(20);
sheet.setDefaultColumnWidth(20);
// 设置样式策略
List<CellStyle> cellStyleList = new ArrayList<>();
cellStyleList.add(style);
cellStyleList.add(style2);
cellStyleList.add(style3);
SimpleVerticalCellStyleStrategy styleStrategy = new SimpleVerticalCellStyleStrategy(new SimpleRowHeightStyleStrategy(20), new SimpleRowWidthStyleStrategy(20), cellStyleList);
// 写入Excel文件
EasyExcel.write(new FileOutputStream("example.xlsx"))
.registerWriteHandler(styleStrategy)
.sheet("Sheet1")
.doWrite(null);
// 关闭工作簿
workbook.close();
}
}
```
注意:在使用自定义颜色之前,需要将颜色添加到调色板中。可以通过`IndexedColors.addCustomColor()`方法将颜色添加到调色板中。另外,EasyExcel提供了一些样式策略,可以轻松实现设置行高、列宽和单元格样式。在本示例中,使用了`SimpleVerticalCellStyleStrategy`来设置单元格样式。
相关推荐
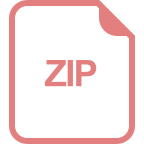
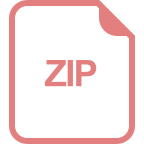














