函数max(int x[],int n)的功能是求有n个元素的数组x中的最大值。主函数中随机输入10个数并保存在数组a中,调用max函数查找数组a中的最大值并输出。请完善该程序。
时间: 2024-02-20 17:01:27 浏览: 192
以下是完整程序的代码:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
int max(int x[], int n);
int main() {
const int N = 10;
int a[N];
srand(time(0)); // 用当前时间作为随机数生成器的种子,确保每次运行程序时生成的随机数是不同的
for (int i = 0; i < N; i++) {
a[i] = rand() % 100; // 生成0到99之间的随机数
cout << a[i] << " ";
}
cout << endl;
cout << "The max number is: " << max(a, N) << endl;
return 0;
}
int max(int x[], int n) {
int max_num = x[0];
for (int i = 1; i < n; i++) {
if (x[i] > max_num) {
max_num = x[i];
}
}
return max_num;
}
```
程序流程如下:
1. 定义一个常量N为10,表示数组元素的个数;
2. 声明一个大小为N的数组a;
3. 用`srand(time(0))`设置随机数生成器的种子,确保每次运行程序时生成的随机数是不同的;
4. 用`for`循环生成N个0到99之间的随机数,并保存在数组a中;
5. 用`for`循环输出数组a中的元素;
6. 调用`max`函数查找数组a中的最大值;
7. 输出最大值。
函数`max`的实现过程如下:
1. 定义一个变量`max_num`,用来保存最大值,初值为数组第一个元素;
2. 用`for`循环遍历数组中的每个元素;
3. 如果当前元素比`max_num`大,则将`max_num`更新为当前元素;
4. 循环结束后,`max_num`即为数组中的最大值,返回`max_num`。
阅读全文
相关推荐
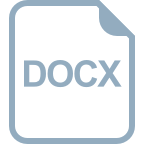
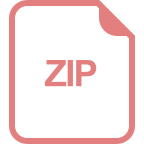
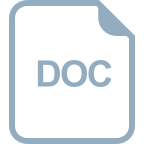















