Write a program to prompt the user to enter a set of strings and the program would sort and display them in ascending alphabetical order. It is accepted to use the functions in the string.h library in the program.
时间: 2024-03-17 16:44:58 浏览: 27
Sure, here's a C program that prompts the user to enter a set of strings, sorts them using the `qsort()` function from the `stdlib.h` library, and then displays them in ascending alphabetical order:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STRINGS 100
#define MAX_STRING_LENGTH 100
int compare_strings(const void* a, const void* b) {
return strcmp(*(const char**)a, *(const char**)b);
}
int main() {
char strings[MAX_STRINGS][MAX_STRING_LENGTH];
int num_strings = 0;
printf("Enter a set of strings (up to %d, one per line, press Ctrl+D to stop):\n", MAX_STRINGS);
// Read in the strings
while (num_strings < MAX_STRINGS && fgets(strings[num_strings], MAX_STRING_LENGTH, stdin)) {
// Remove the newline character at the end
strings[num_strings][strcspn(strings[num_strings], "\n")] = '\0';
num_strings++;
}
// Sort the strings
qsort(strings, num_strings, sizeof(char[MAX_STRING_LENGTH]), compare_strings);
// Display the sorted strings
printf("Sorted strings:\n");
for (int i = 0; i < num_strings; i++) {
printf("%s\n", strings[i]);
}
return 0;
}
```
In this program, the `compare_strings()` function is used as the comparison function for `qsort()`, which takes two `const void*` arguments and returns an integer indicating the relative order of the two elements. The `main()` function prompts the user to enter a set of strings, reads them in using `fgets()`, sorts them using `qsort()`, and then displays them using `printf()`.
相关推荐
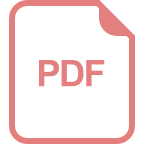
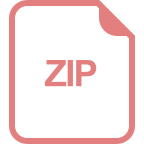













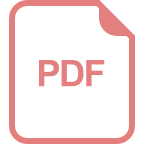
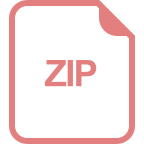