用定时器将L2,L4,L5,L6间隔一秒闪烁
时间: 2024-10-28 16:06:17 浏览: 36
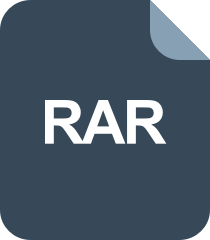
8051单片机的定时器/计数器
要使用定时器让L2、L4、L5和L6这四个LED灯每隔一秒轮流闪烁,通常是在电子电路设计或微控制器编程环境中实现。这里假设你在编写Arduino或类似平台的代码,可以按照以下步骤操作:
1. 首先,导入必要的库,如`SoftwareSerial`(如果用于串口通信)和`TimerOne`。
```c++
#include <TimerOne.h>
```
2. 定义一个数组来存储LED的状态,初始化它们为非亮状态。
```c++
int ledState[4] = {0, 0, 0, 0};
```
3. 设置定时器1,设置其分频系数,以便1秒后触发中断。
```c++
void setup() {
Timer1.initialize(1000); // 1000 for a 1-second interval (adjust according to your system)
}
```
4. 创建一个中断服务程序(ISR),在这个函数里,改变LED的状态并循环处理下一个LED。
```c++
void timerISR() {
static int currentLed = 0;
ledState[currentLed] = !ledState[currentLed]; // Toggle the state of the LED
if (currentLed == 3) {
currentLed = 0; // Start from the first LED again after L6
} else {
currentLed++;
}
// Update the LED connected to the appropriate pin based on the state
digitalWrite(L2, ledState[0]);
digitalWrite(L4, ledState[1]);
digitalWrite(L5, ledState[2]);
digitalWrite(L6, ledState[3]);
}
void loop() {
attachInterrupt(digitalPinToInterrupt(TIMER1_PIN), timerISR, RISING); // Attach interrupt to Timer1's rising edge
}
```
5. 确保在`loop()`函数中正确地连接了中断到Timer1的上升沿,替换`TIMER1_PIN`为实际的硬件连接。
这个示例假设每个LED连接到了特定的数字引脚,`L2`, `L4`, `L5`, 和 `L6`。当定时器1中断触发时,它会改变当前LED的状态并切换到下一个。
阅读全文
相关推荐
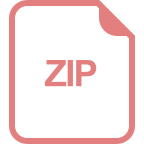
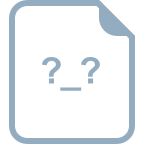














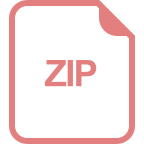
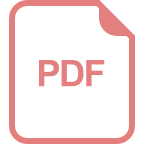