请用python tensorflow写出一个物体识别分类代码,包括下载数据、训练、从摄像头读入图像并实时推理。要求代码运行后可以直接从下载数据、训练,一直到推理。
时间: 2023-09-10 11:05:31 浏览: 126
### 回答1:
下面是一个使用 TensorFlow 实现物体识别分类的示例代码,包括从摄像头读入图像并进行实时推理的部分。这份代码需要您自己下载数据集并进行训练。
首先,需要导入所需的库和模块:
```python
import tensorflow as tf
import numpy as np
import cv2
# 下载数据集(需要自己实现)
# dataset = download_dataset()
# 训练模型(需要自己实现)
# model = train(dataset)
# 定义摄像头输入的大小
camera_input_size = (224, 224)
# 打开摄像头
camera = cv2.VideoCapture(0)
# 不断循环,读取摄像头的输入帧并进行推理
while True:
# 读取一帧图像
_, frame = camera.read()
# 将图像缩放到模型输入所需的大小
frame = cv2.resize(frame, camera_input_size)
frame = np.expand_dims(frame, axis=0)
frame = frame.astype(np.float32)
# 进行推理
predictions = model(frame)
# 将结果转化为概率最大的分类
label = np.argmax(predictions)
# 在图像上绘制分类的标签
cv2.putText(frame, label, (10, 10), cv2.FONT_HERSHEY_SIMPLEX, 1, (255, 255, 255))
# 展示图像
cv2.imshow("Frame", frame)
# 等待按键输入,如果按下 q 键则退出
key = cv2.waitKey(1)
if key == ord("q"):
break
# 关闭
### 回答2:
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras import datasets, layers, models
# 下载数据
(train_images, train_labels), (test_images, test_labels) = datasets.cifar10.load_data()
train_images, test_images = train_images / 255.0, test_images / 255.0
# 训练
model = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(10)
])
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=10, validation_data=(test_images, test_labels))
# 从摄像头读入图像并实时推理
import cv2
class_names = ['airplane', 'automobile', 'bird', 'cat', 'deer', 'dog', 'frog', 'horse', 'ship', 'truck']
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
frame = cv2.resize(frame, (32, 32))
frame = frame / 255.0
predictions = model.predict(tf.expand_dims(frame, axis=0))
score = tf.nn.softmax(predictions[0])
predicted_class = class_names[tf.argmax(score)]
confidence = round(100 * tf.reduce_max(score).numpy(), 2)
cv2.putText(frame, f"{predicted_class} {confidence}%", (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0, 255, 0), 2)
cv2.imshow('Object Recognition', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
### 回答3:
下面是一个使用Python和TensorFlow编写的物体识别分类代码的示例,包括下载数据、训练和实时推理功能。
```python
import tensorflow as tf
from tensorflow.keras.datasets import cifar10
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Dense, Flatten
from tensorflow.keras.utils import to_categorical
import cv2
# 下载和准备数据
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
x_train = x_train / 255.0
x_test = x_test / 255.0
y_train = to_categorical(y_train)
y_test = to_categorical(y_test)
# 构建模型
model = Sequential()
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)))
model.add(MaxPooling2D((2, 2)))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D((2, 2)))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(Flatten())
model.add(Dense(64, activation='relu'))
model.add(Dense(10, activation='softmax'))
# 编译和训练模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test))
# 从摄像头读取图像并实时进行推理
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
frame = cv2.resize(frame, (32, 32))
frame = frame.astype('float32') / 255.0
frame = tf.expand_dims(frame, axis=0)
prediction = model.predict(frame)
class_index = tf.argmax(prediction, axis=1)
class_name = ['airplane', 'automobile', 'bird', 'cat', 'deer', 'dog', 'frog', 'horse', 'ship', 'truck'][int(class_index)]
cv2.putText(frame, class_name, (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
cv2.imshow('Object Detection', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
注意,该示例代码假设您已经安装了TensorFlow、OpenCV和其他必要的库。执行代码后,它将下载CIFAR-10数据集,使用该数据集训练一个简单的卷积神经网络模型,并从摄像头读取图像并进行实时推理。在窗口中,它会显示识别出的物体类别。使用此代码,您可以直接从下载数据、训练到推理整个过程。
阅读全文
相关推荐
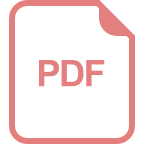
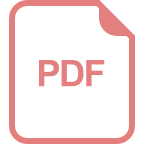
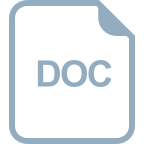
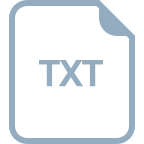
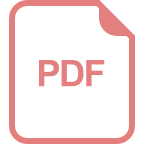
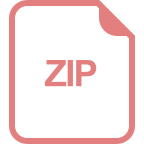
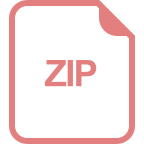
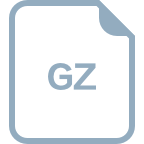
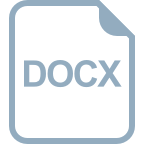
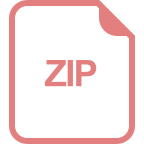
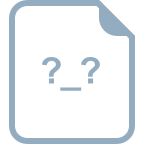
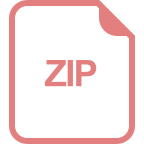
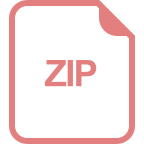
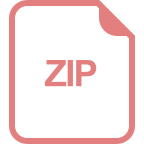
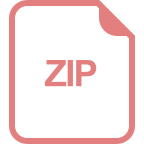
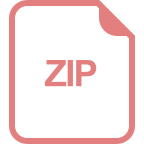
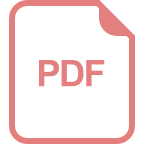