opencv手势识别python
时间: 2023-09-09 22:10:25 浏览: 63
OpenCV是一个非常流行的计算机视觉库,可以用来进行图像处理、特征提取、对象检测等任务。手势识别是计算机视觉领域的一个重要研究方向之一,OpenCV也提供了一些功能来实现手势识别。
在Python中使用OpenCV进行手势识别的步骤如下:
1. 安装OpenCV库
```
pip install opencv-python
```
2. 导入OpenCV库
```
import cv2
```
3. 加载图像
```
img = cv2.imread('hand.jpg')
```
4. 转换图像为灰度图
```
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
5. 进行图像的二值化
```
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
```
6. 进行轮廓检测
```
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
```
7. 找到手掌轮廓
```
hand_contour = max(contours, key=cv2.contourArea)
```
8. 找到手指轮廓
```
finger_contours = []
for contour in contours:
if cv2.contourArea(contour) > 1000 and cv2.contourArea(contour) < cv2.contourArea(hand_contour):
finger_contours.append(contour)
```
9. 判断手指数量
```
num_fingers = len(finger_contours)
```
10. 显示结果
```
cv2.drawContours(img, [hand_contour], 0, (0, 255, 0), 2)
cv2.drawContours(img, finger_contours, -1, (0, 0, 255), 2)
cv2.putText(img, str(num_fingers), (50, 50), cv2.FONT_HERSHEY_SIMPLEX, 2, (0, 0, 255), 2)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
以上就是使用OpenCV进行手势识别的Python代码示例。需要注意的是,这只是一个简单的示例,实际应用中需要根据具体情况进行调整和优化。
相关推荐
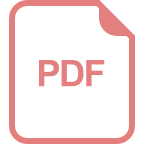
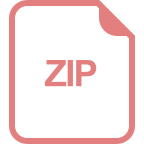













