定义一个计数器类counter,包含私有成员int n,重载运算符“+”
时间: 2023-05-29 07:04:06 浏览: 322
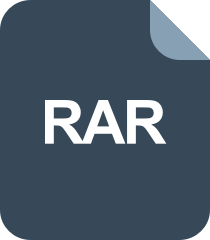
枚举,“+”号重载,源代码

,使得计数器对象可以像整数一样进行加法和减法操作。
以下是可能的实现:
```c++
class Counter {
private:
int n;
public:
Counter(int n = 0) : n(n) {}
// 重载运算符+
Counter operator+(const Counter& other) const {
return Counter(n + other.n);
}
// 重载运算符-
Counter operator-(const Counter& other) const {
return Counter(n - other.n);
}
// 重载运算符+=
Counter& operator+=(const Counter& other) {
n += other.n;
return *this;
}
// 重载运算符-=
Counter& operator-=(const Counter& other) {
n -= other.n;
return *this;
}
// 重载运算符++
Counter& operator++() {
++n;
return *this;
}
// 重载运算符--
Counter& operator--() {
--n;
return *this;
}
// 重载运算符<<
friend std::ostream& operator<<(std::ostream& os, const Counter& counter) {
os << counter.n;
return os;
}
};
```
使用示例:
```c++
Counter c1(10);
Counter c2(5);
Counter c3 = c1 + c2; // 等价于 Counter c3 = c1.operator+(c2);
std::cout << c3 << std::endl; // 输出 15
Counter c4 = c1 - c2; // 等价于 Counter c4 = c1.operator-(c2);
std::cout << c4 << std::endl; // 输出 5
c1 += c2; // 等价于 c1.operator+=(c2);
std::cout << c1 << std::endl; // 输出 15
c1 -= c2; // 等价于 c1.operator-=(c2);
std::cout << c1 << std::endl; // 输出 10
++c1; // 等价于 c1.operator++();
std::cout << c1 << std::endl; // 输出 11
--c1; // 等价于 c1.operator--();
std::cout << c1 << std::endl; // 输出 10
```
阅读全文
相关推荐














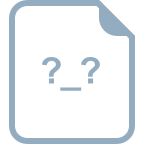