uniapp nvue methods
时间: 2023-08-12 12:21:19 浏览: 43
在 nvue 中,methods 是一个用于存放组件中方法的对象。这个对象中的每一个属性都是一个方法,可以在组件中通过 this 调用。例如:
```
<template>
<view>
<button @click="handleClick">点击我</button>
</view>
</template>
<script>
export default {
methods: {
handleClick() {
console.log('点击了按钮')
}
}
}
</script>
```
在上面的代码中,我们在 methods 对象中定义了一个名为 handleClick 的方法,然后在模板中通过 @click 绑定到了一个按钮上。当按钮被点击时,handleClick 方法就会被调用。需要注意的是,methods 中的方法都是属于组件实例的,可以通过 this 调用组件中的其它属性和方法。
相关问题
uniapp nvue、vue的webview实现播放视频全屏
对于uni-app的nvue,可以使用uni-app提供的`uni-video`组件来实现视频播放,同时支持全屏模式。在`uni-video`组件中,设置`show-fullscreen-btn`为true,即可显示全屏按钮,点击全屏按钮后,视频会自动全屏。
对于vue的webview实现播放视频全屏,可以使用HTML5的Video标签,并且在进入全屏模式时,需要调用浏览器提供的API。具体实现步骤如下:
1. 在Vue模板中,使用Video标签来播放视频,并设置控制栏为浮动状态。
```html
<video
src="your_video_file.mp4"
controls
style="position:fixed; top:0; left:0; width:100%; height:100%; z-index:9999;"
></video>
```
2. 在Vue方法中,监听全屏事件,并在全屏时调用浏览器提供的API。
```javascript
methods: {
enterFullScreen() {
var video = document.getElementsByTagName('video')[0];
if (video.requestFullscreen) {
video.requestFullscreen();
} else if (video.mozRequestFullScreen) {
video.mozRequestFullScreen();
} else if (video.webkitRequestFullscreen) {
video.webkitRequestFullscreen();
}
}
}
```
3. 在控制栏中添加全屏按钮,并在点击时调用Vue方法。
```html
<div class="controls">
<button @click="enterFullScreen">全屏</button>
</div>
```
4. 最后,需要在Vue的生命周期中监听浏览器的全屏事件,并在退出全屏时恢复控制栏的位置。
```javascript
mounted() {
document.addEventListener('fullscreenchange', this.exitFullScreen);
document.addEventListener('webkitfullscreenchange', this.exitFullScreen);
document.addEventListener('mozfullscreenchange', this.exitFullScreen);
document.addEventListener('MSFullscreenChange', this.exitFullScreen);
},
exitFullScreen() {
var video = document.getElementsByTagName('video')[0];
if (!document.fullscreenElement && !document.webkitFullscreenElement && !document.mozFullScreenElement && !document.msFullscreenElement) {
video.style = "position:fixed; top:50%; left:50%; transform:translate(-50%, -50%);";
}
}
```
uniapp中nvue里怎么压缩视频
在uni-app的nvue中,可以使用uniMediaPicker组件选择视频文件,然后使用uniCloud云函数或者后端服务来压缩视频。下面是一个简单的示例:
1. 在nvue页面中引入uniMediaPicker组件:
```html
<template>
<view>
<button @click="chooseVideo">选择视频</button>
</view>
</template>
<script>
import { uniMediaPicker } from '@dcloudio/uni-ui'
export default {
methods: {
chooseVideo() {
uniMediaPicker({
mediaType: 2, // 视频类型
success: (res) => {
const tempFilePath = res.tempFiles[0].path
this.compressVideo(tempFilePath)
}
})
},
compressVideo(path) {
// 调用uniCloud云函数或者后端服务进行视频压缩
// 在云函数中使用ffmpeg或其他视频处理库来压缩视频
// 返回压缩后的视频路径或者数据流给前端
}
}
}
</script>
```
2. 在云函数或者后端服务中使用视频处理库(如ffmpeg)来进行视频压缩。具体的压缩方式和参数可以根据需要进行调整。
3. 将压缩后的视频路径或者数据流返回给前端,然后根据需要进行展示或者保存等操作。
请注意,视频压缩是一个相对复杂的操作,需要对视频编解码有一定的了解。建议参考相关的文档和示例代码来完成视频压缩的具体实现。
相关推荐
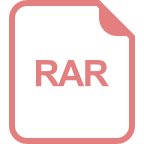












