写一个Android上面使用libyuv库对1920x1080的nv12数据分别旋转y通道和uv通道90度的示例代码
时间: 2024-01-21 16:16:53 浏览: 23
以下是一个使用libyuv库对1920x1080的nv12数据分别旋转y通道和uv通道90度的示例代码:
```java
import org.webrtc.NV21Buffer;
import org.webrtc.VideoFrame;
import org.webrtc.VideoFrame.Buffer;
public class YUVUtils {
// 旋转y通道90度
public static byte[] rotateY(byte[] yData, int width, int height) {
byte[] rotatedY = new byte[width * height];
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
rotatedY[j * height + height - i - 1] = yData[i * width + j];
}
}
return rotatedY;
}
// 旋转uv通道90度
public static byte[] rotateUV(byte[] uvData, int width, int height) {
byte[] rotatedUV = new byte[width * height / 2];
for (int i = 0; i < height / 2; i++) {
for (int j = 0; j < width / 2; j++) {
rotatedUV[j * height / 2 + height / 2 - i - 1] = uvData[i * width + j * 2];
rotatedUV[j * height / 2 + height / 2 + width / 2 - i - 1] = uvData[i * width + j * 2 + 1];
}
}
return rotatedUV;
}
// 旋转nv12数据的y和uv通道90度
public static byte[] rotateNV12(byte[] nv12Data, int width, int height) {
byte[] rotatedY = rotateY(nv12Data, width, height);
byte[] rotatedUV = rotateUV(nv12Data, width, height);
byte[] rotatedNV12 = new byte[width * height * 3 / 2];
System.arraycopy(rotatedY, 0, rotatedNV12, 0, width * height);
System.arraycopy(rotatedUV, 0, rotatedNV12, width * height, width * height / 2);
return rotatedNV12;
}
}
// 在使用时,可以将nv12数据转换为VideoFrame.Buffer,然后通过libyuv库进行旋转
byte[] nv12Data = ...
NV21Buffer buffer = new NV21Buffer(nv12Data, width, height, null);
VideoFrame.I420Buffer i420Buffer = buffer.toI420();
byte[] rotatedNV12 = YUVUtils.rotateNV12(i420Buffer.getDataY(), width, height);
VideoFrame.Buffer rotatedBuffer = new NV21Buffer(rotatedNV12, height, width, null);
```
相关推荐
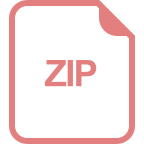














