张量下使用指定卷积核([0, 1, 0], [1, -4, 1], [0, 1, 0])的深度卷积pytorch代码
时间: 2023-10-01 12:09:20 浏览: 121
以下是一个使用指定卷积核进行深度卷积的PyTorch代码示例:
```python
import torch
import torch.nn as nn
# 定义深度卷积的卷积核
kernel = torch.tensor([[0, 1, 0], [1, -4, 1], [0, 1, 0]], dtype=torch.float32)
# 定义深度卷积的卷积层
conv_layer = nn.Conv2d(in_channels=3, out_channels=6, kernel_size=3, padding=1, bias=False)
# 将卷积核复制到每个通道上
weights = kernel.repeat((6, 3, 1, 1))
# 将卷积核设置为不可训练
conv_layer.weight = nn.Parameter(weights, requires_grad=False)
# 定义一个随机输入张量
x = torch.randn(1, 3, 32, 32)
# 对输入张量进行深度卷积
output = conv_layer(x)
# 打印输出张量的形状
print(output.shape)
```
在这个示例中,我们首先定义了一个3×3的卷积核,然后定义了一个卷积层,该卷积层有6个输出通道。我们将卷积核复制到每个通道上,并将其设置为不可训练。最后,我们将一个随机输入张量传递给卷积层,并打印输出张量的形状。
相关问题
张量下指定卷积核为([0, 1, 0], [1, -4, 1], [0, 1, 0])的depthwise convolution 的 PyTorch 代码
```python
import torch
import torch.nn as nn
# 创建输入张量
input_tensor = torch.randn(1, 3, 5, 5)
# 定义depthwise convolution层
depthwise_conv = nn.Conv2d(in_channels=3, out_channels=3, kernel_size=3, stride=1, padding=1, groups=3, bias=False)
# 指定卷积核为([0, 1, 0], [1, -4, 1], [0, 1, 0])
depthwise_conv.weight.data = torch.tensor([[[[0, 1, 0], [1, -4, 1], [0, 1, 0]]],
[[[0, 1, 0], [1, -4, 1], [0, 1, 0]]],
[[[0, 1, 0], [1, -4, 1], [0, 1, 0]]]], dtype=torch.float)
# 对输入张量进行depthwise convolution操作
output_tensor = depthwise_conv(input_tensor)
print(output_tensor.shape) # (1, 3, 5, 5)
```
张量中指定卷积核为[[0, 1, 0], [1, -4, 1], [0, 1, 0]]的卷积代码pytorch
import torch.nn.functional as F
# assuming input_tensor is the input tensor to be convolved
kernel = torch.tensor([[0, 1, 0], [1, -4, 1], [0, 1, 0]], dtype=torch.float32)
input_tensor = input_tensor.unsqueeze(0).unsqueeze(0) # add batch and channel dimension
output_tensor = F.conv2d(input_tensor, kernel.unsqueeze(0).unsqueeze(0)) # convolve the tensor with the kernel
output_tensor = output_tensor.squeeze() # remove batch and channel dimension
阅读全文
相关推荐
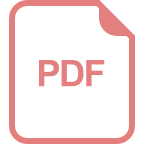
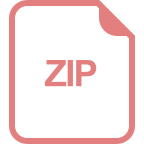






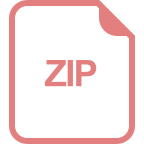
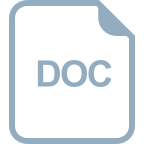






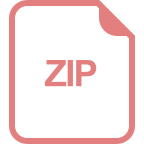