java中接口的所有功能以及示例
时间: 2023-05-31 22:03:27 浏览: 94
接口是Java中一种特殊的抽象类,它定义了一组方法的规范,但没有提供实现。接口可以被多个类实现,使得这些类具有相同的行为规范。在Java中,接口被用于实现多态性和代码复用。
下面是Java中接口的所有功能:
1. 定义接口
接口可以通过interface关键字定义,如下所示:
```
public interface MyInterface {
void myMethod();
}
```
2. 实现接口
类可以通过implements关键字实现接口,如下所示:
```
public class MyClass implements MyInterface {
public void myMethod() {
System.out.println("MyClass implements MyInterface");
}
}
```
3. 多继承接口
类可以同时实现多个接口,如下所示:
```
public class MyClass implements MyInterface1, MyInterface2 {
public void myMethod1() {
System.out.println("MyClass implements MyInterface1");
}
public void myMethod2() {
System.out.println("MyClass implements MyInterface2");
}
}
```
4. 接口继承接口
接口可以通过extends关键字继承接口,如下所示:
```
public interface MyInterface2 extends MyInterface1 {
void myMethod2();
}
```
5. 默认方法
接口可以提供默认方法,如下所示:
```
public interface MyInterface {
default void myMethod() {
System.out.println("Default Method in MyInterface");
}
}
```
6. 静态方法
接口可以提供静态方法,如下所示:
```
public interface MyInterface {
static void myStaticMethod() {
System.out.println("Static Method in MyInterface");
}
}
```
7. 常量
接口可以定义常量,如下所示:
```
public interface MyInterface {
int MY_CONSTANT = 100;
}
```
示例:
```
public interface Shape {
double getArea(); // 计算面积
double getPerimeter(); // 计算周长
}
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
public double getPerimeter() {
return 2 * Math.PI * radius;
}
}
public class Rectangle implements Shape {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double getArea() {
return length * width;
}
public double getPerimeter() {
return 2 * (length + width);
}
}
public class Main {
public static void main(String[] args) {
Shape circle = new Circle(5);
Shape rectangle = new Rectangle(4, 6);
System.out.println("Circle Area: " + circle.getArea());
System.out.println("Circle Perimeter: " + circle.getPerimeter());
System.out.println("Rectangle Area: " + rectangle.getArea());
System.out.println("Rectangle Perimeter: " + rectangle.getPerimeter());
}
}
```
阅读全文
相关推荐






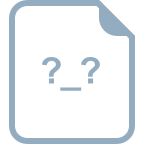


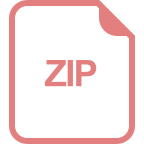

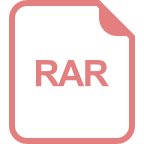


