Java 读取两个excel表并进行每一行的数据比较,不相同的列输出
时间: 2024-02-21 22:57:06 浏览: 22
你可以使用Apache POI库来读取Excel文件,并使用Java中的集合来比较两个表中的数据。以下是一个简单的示例代码,它可以读取两个名为“table1.xlsx”和“table2.xlsx”的Excel文件,并比较每行中的数据。如果两行数据不相同,它将输出该行的列值。
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class ExcelComparator {
public static void main(String[] args) throws IOException {
String file1 = "table1.xlsx";
String file2 = "table2.xlsx";
List<Map<String, String>> table1 = readExcel(file1);
List<Map<String, String>> table2 = readExcel(file2);
// Compare two tables
for (int i = 0; i < table1.size(); i++) {
Map<String, String> row1 = table1.get(i);
Map<String, String> row2 = table2.get(i);
for (Map.Entry<String, String> entry : row1.entrySet()) {
String columnName = entry.getKey();
String value1 = entry.getValue();
String value2 = row2.get(columnName);
if (!value1.equals(value2)) {
System.out.println("Different values in Row " + (i+1) + ", Column " + columnName + ": " + value1 + " " + value2);
}
}
}
}
private static List<Map<String, String>> readExcel(String filename) throws IOException {
List<Map<String, String>> table = new ArrayList<>();
File excelFile = new File(filename);
FileInputStream inputStream = new FileInputStream(excelFile);
Workbook workbook = WorkbookFactory.create(inputStream);
Sheet sheet = workbook.getSheetAt(0);
Row headerRow = sheet.getRow(0);
List<String> columnNames = new ArrayList<>();
for (int i = 0; i < headerRow.getLastCellNum(); i++) {
Cell cell = headerRow.getCell(i);
columnNames.add(cell.getStringCellValue());
}
for (int i = 1; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
Map<String, String> rowData = new HashMap<>();
for (int j = 0; j < columnNames.size(); j++) {
Cell cell = row.getCell(j);
String value = "";
if (cell != null) {
switch (cell.getCellType()) {
case STRING:
value = cell.getStringCellValue();
break;
case NUMERIC:
value = String.valueOf(cell.getNumericCellValue());
break;
case BOOLEAN:
value = String.valueOf(cell.getBooleanCellValue());
break;
default:
value = "";
}
}
rowData.put(columnNames.get(j), value);
}
table.add(rowData);
}
inputStream.close();
return table;
}
}
```
请注意,此示例代码假定两个Excel文件具有相同的列名和相同的行数。如果两个文件不匹配,代码可能会抛出异常或输出错误的结果。你需要自己根据实际情况进行修改和优化。
相关推荐
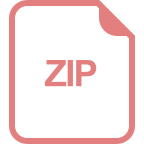
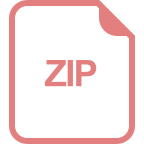














