python aes加密解密(不加库)
时间: 2023-06-22 13:40:11 浏览: 194
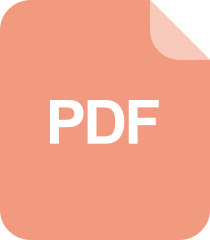
python实现AES加密解密
好的,以下是Python中使用AES加密解密的示例代码(不使用任何第三方库):
```python
import base64
import hashlib
from Crypto import Random
from Crypto.Cipher import AES
class AESCipher:
def __init__(self, key):
self.key = hashlib.sha256(key.encode()).digest()
def encrypt(self, plaintext):
iv = Random.new().read(AES.block_size)
cipher = AES.new(self.key, AES.MODE_CBC, iv)
ciphertext = cipher.encrypt(self._pad(plaintext).encode())
return base64.b64encode(iv + ciphertext).decode()
def decrypt(self, ciphertext):
ciphertext = base64.b64decode(ciphertext)
iv = ciphertext[:AES.block_size]
cipher = AES.new(self.key, AES.MODE_CBC, iv)
plaintext = self._unpad(cipher.decrypt(ciphertext[AES.block_size:])).decode()
return plaintext
def _pad(self, s):
padding_size = AES.block_size - len(s) % AES.block_size
padding = chr(padding_size) * padding_size
return s + padding
@staticmethod
def _unpad(s):
padding_size = ord(s[-1])
return s[:-padding_size]
```
使用示例:
```python
ciphertext = AESCipher('my_key').encrypt('Hello World!')
print(ciphertext) # 输出类似:'fXpNjJ9+1KPQJnKbSj2l2A=='
plaintext = AESCipher('my_key').decrypt(ciphertext)
print(plaintext) # 输出:'Hello World!'
```
请注意,加密和解密时使用相同的密钥。可以将密钥设置为任何字符串,但最好使用足够长的字符串并使用哈希函数进行处理,以增加安全性。
阅读全文
相关推荐
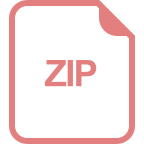




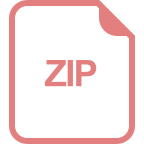
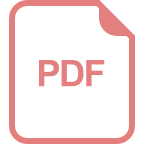
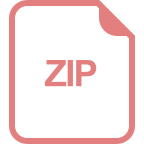
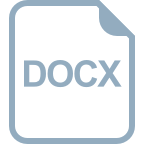