qt linux读写io口
时间: 2023-07-08 22:07:27 浏览: 52
在 Linux 上,可以通过文件系统访问 I/O 端口,但需要使用特定的设备驱动程序。在 Qt 中,可以通过 QIODevice 类来访问设备驱动程序。
以下是一个使用 QIODevice 类读写 I/O 端口的示例代码:
```cpp
#include <QtCore>
#include <sys/io.h>
class IoDevice : public QIODevice
{
public:
IoDevice(quint16 port, QObject* parent = nullptr) : QIODevice(parent), m_port(port)
{
if (ioperm(m_port, 1, 1) != 0) {
qFatal("ioperm failed");
exit(1);
}
}
~IoDevice()
{
ioperm(m_port, 1, 0);
}
protected:
qint64 readData(char* data, qint64 maxSize) override
{
unsigned char ch = inb(m_port);
*data = ch;
return 1;
}
qint64 writeData(const char* data, qint64 maxSize) override
{
unsigned char ch = *data;
outb(ch, m_port);
return 1;
}
private:
quint16 m_port;
};
int main(int argc, char** argv)
{
QCoreApplication app(argc, argv);
IoDevice ioDevice(0x378);
if (!ioDevice.open(QIODevice::ReadWrite)) {
qFatal("Failed to open I/O device");
exit(1);
}
char data;
if (ioDevice.read(&data, 1) == 1) {
qDebug() << "Read data:" << data;
}
char sendData = 0x55;
if (ioDevice.write(&sendData, 1) == 1) {
qDebug() << "Write data:" << sendData;
}
ioDevice.close();
return app.exec();
}
```
上述示例代码中,我们创建了一个名为 `IoDevice` 的自定义 `QIODevice` 类。在构造函数中,我们使用 `ioperm` 函数请求访问 I/O 端口。在析构函数中,我们释放了对 I/O 端口的访问。在 `readData` 和 `writeData` 函数中,我们分别使用 `inb` 和 `outb` 函数读写 I/O 端口。在 `main` 函数中,我们创建了 `IoDevice` 类的实例,并使用 `open` 函数打开 I/O 端口。然后,我们使用 `read` 函数读取一个字节的数据,并使用 `write` 函数写入一个字节的数据。最后,我们关闭了 `IoDevice` 类的实例。
需要注意的是,访问 I/O 端口需要特权级别较高的权限,因此需要使用 root 权限运行程序。
相关推荐
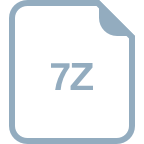














