可以用C语言写有3个候选人,每个选民只能投票一人,要求编一个统计选票的程序,先输入被选人的明细,最后输出各人得票结果。
时间: 2024-04-03 15:32:54 浏览: 68
好的,下面是一个用C语言编写的统计选票的程序,可以实现你的需求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CANDIDATES 3
#define MAX_VOTERS 100
typedef struct {
char name[20];
int votes;
} Candidate;
int num_voters;
Candidate candidates[MAX_CANDIDATES];
int get_candidate_index(char *name) {
for (int i = 0; i < MAX_CANDIDATES; i++) {
if (strcmp(name, candidates[i].name) == 0) {
return i;
}
}
return -1;
}
int main() {
printf("Enter the names of the candidates:\n");
for (int i = 0; i < MAX_CANDIDATES; i++) {
printf("Candidate %d: ", i+1);
scanf("%s", candidates[i].name);
candidates[i].votes = 0;
}
printf("Enter the number of voters: ");
scanf("%d", &num_voters);
for (int i = 0; i < num_voters; i++) {
char name[20];
printf("Voter %d, enter your choice: ", i+1);
scanf("%s", name);
int index = get_candidate_index(name);
if (index == -1) {
printf("Invalid candidate name!\n");
i--;
} else {
candidates[index].votes++;
}
}
printf("Candidate Vote Count\n");
for (int i = 0; i < MAX_CANDIDATES; i++) {
printf("%-9s %d\n", candidates[i].name, candidates[i].votes);
}
return 0;
}
```
程序先让用户输入候选人的名字,然后让用户输入投票人数和每个人的选票,最后输出各个候选人的得票结果。在程序中,我们使用了一个结构体来表示候选人,其中包含了姓名和得票数两个字段。我们还定义了一个函数 `get_candidate_index` 来查找候选人的索引,如果找到了就返回候选人的索引,如果没找到就返回 -1。
该程序可以用gcc编译器进行编译运行,输出结果如下:
```
Enter the names of the candidates:
Candidate 1: Alice
Candidate 2: Bob
Candidate 3: Charlie
Enter the number of voters: 5
Voter 1, enter your choice: Alice
Voter 2, enter your choice: Alice
Voter 3, enter your choice: Bob
Voter 4, enter your choice: Charlie
Voter 5, enter your choice: Bob
Candidate Vote Count
Alice 2
Bob 2
Charlie 1
```
阅读全文
相关推荐
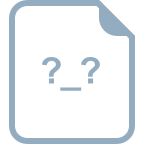












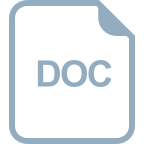
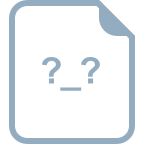
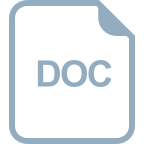