图书馆管理系统 Java代码
时间: 2023-10-29 12:07:15 浏览: 30
以下是一个简单的图书馆管理系统的 Java 代码示例:
```
import java.util.ArrayList;
import java.util.Scanner;
public class LibrarySystem {
private static ArrayList<Book> bookList = new ArrayList<>();
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int choice;
do {
System.out.println("1. Add Book");
System.out.println("2. Display All Books");
System.out.println("3. Search Book");
System.out.println("4. Delete Book");
System.out.println("5. Exit");
System.out.print("Enter your choice: ");
choice = scanner.nextInt();
switch (choice) {
case 1:
addBook();
break;
case 2:
displayAllBooks();
break;
case 3:
searchBook();
break;
case 4:
deleteBook();
break;
case 5:
System.out.println("Exiting Library System...");
break;
default:
System.out.println("Invalid choice. Please try again.");
}
} while (choice != 5);
}
private static void addBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter book title: ");
String title = scanner.nextLine();
System.out.print("Enter book author: ");
String author = scanner.nextLine();
System.out.print("Enter book ISBN: ");
String isbn = scanner.nextLine();
System.out.print("Enter book year: ");
int year = scanner.nextInt();
Book book = new Book(title, author, isbn, year);
bookList.add(book);
System.out.println("Book added successfully.");
}
private static void displayAllBooks() {
if (bookList.size() == 0) {
System.out.println("No books found.");
} else {
System.out.println("Books in the library:");
for (Book book : bookList) {
System.out.println(book);
}
}
}
private static void searchBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter book title or author: ");
String query = scanner.nextLine();
ArrayList<Book> results = new ArrayList<>();
for (Book book : bookList) {
if (book.getTitle().contains(query) || book.getAuthor().contains(query)) {
results.add(book);
}
}
if (results.size() == 0) {
System.out.println("No results found.");
} else {
System.out.println("Results for \"" + query + "\":");
for (Book book : results) {
System.out.println(book);
}
}
}
private static void deleteBook() {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter book title or author: ");
String query = scanner.nextLine();
ArrayList<Book> results = new ArrayList<>();
for (Book book : bookList) {
if (book.getTitle().contains(query) || book.getAuthor().contains(query)) {
results.add(book);
}
}
if (results.size() == 0) {
System.out.println("No results found.");
} else {
System.out.println("Results for \"" + query + "\":");
for (int i = 0; i < results.size(); i++) {
Book book = results.get(i);
System.out.println((i + 1) + ". " + book);
}
System.out.print("Enter the number of the book to delete: ");
int index = scanner.nextInt() - 1;
bookList.remove(results.get(index));
System.out.println("Book deleted successfully.");
}
}
}
class Book {
private String title;
private String author;
private String isbn;
private int year;
public Book(String title, String author, String isbn, int year) {
this.title = title;
this.author = author;
this.isbn = isbn;
this.year = year;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getIsbn() {
return isbn;
}
public int getYear() {
return year;
}
@Override
public String toString() {
return title + " by " + author + " (" + year + ")";
}
}
```
这个简单的图书馆管理系统可以让用户添加、显示、搜索和删除图书。图书信息存储在一个 `ArrayList` 中,每本书由 `Book` 类表示。用户可以根据书名或作者搜索图书,并选择要删除的图书。
相关推荐
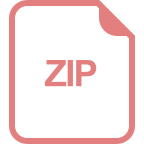
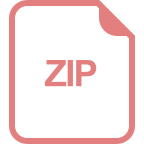














