写出识别c语言标识符和无符号十进制整数的c语言代码段
时间: 2024-09-26 08:11:04 浏览: 63
在C语言中,我们可以使用`ctype.h`库中的函数来检查字符是否属于合法的标识符字符集,同时通过`scanf`函数结合自定义格式转换来识别无符号十进制整数。以下是一个简单的代码片段:
```c
#include <stdio.h>
#include <ctype.h>
int is_identifier_char(char c) {
// 标识符由字母、下划线或数字组成
return (isalnum(c) || c == '_');
}
bool is_valid_identifier(const char* str) {
if (*str == '\0') return true; // 空字符串是有效标识符
for (int i = 0; str[i] != '\0'; ++i) {
if (!is_identifier_char(str[i])) return false;
}
return true;
}
int parse_unsigned_decimal(const char* str) {
int num = 0;
char *endptr;
errno = 0; // 初始化错误标志位
num = strtoul(str, &endptr, 10); // 尝试将字符串解析为无符号长整型
if (errno == ERANGE || endptr == str || *endptr != '\0') { // 错误情况处理
printf("Invalid integer format.\n");
return -1;
} else {
return num;
}
}
int main() {
const char* identifier = "myVariable";
const char* number = "42";
if (is_valid_identifier(identifier)) {
printf("%s is a valid identifier.\n", identifier);
} else {
printf("%s is not a valid identifier.\n", identifier);
}
int parsed_number = parse_unsigned_decimal(number);
if (parsed_number != -1) {
printf("The integer value is %d\n", parsed_number);
} else {
printf("Failed to parse the integer.\n");
}
return 0;
}
```
在这个例子中,`is_identifier_char`用于检查单个字符是否为有效的标识符字符,而`is_valid_identifier`遍历整个字符串判断是否全部符合规则。`parse_unsigned_decimal`函数则尝试将输入的字符串解析成无符号整数。
阅读全文
相关推荐
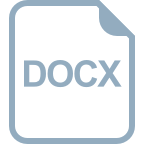
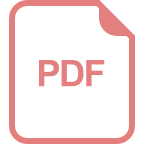
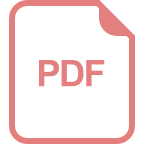















