宿舍管理系统详细设计与具体代码实现爬虫
时间: 2024-11-11 15:12:48 浏览: 19
宿舍管理系统通常是一个用于管理学生住宿信息、分配房间、处理入住退宿等操作的软件系统。其详细设计会包括以下几个关键部分:
1. **需求分析**:明确系统需要管理哪些数据(如学生信息、宿舍信息、床位分配等),以及用户有哪些功能需求(如查询、添加、删除记录等)。
2. **数据库设计**:设计数据库模型,例如创建学生表、宿舍表、床位表等,并确定它们之间的关联。
3. **模块划分**:将系统划分为前端界面、后台服务和数据访问层。前端用于用户交互,后台处理业务逻辑,数据访问层负责与数据库通信。
4. **功能设计**:编写每个功能模块的具体逻辑,比如登录验证、宿舍分配算法、错误处理等。
5. **爬虫实现**(如果涉及到):对于一些外部数据源(如在线宿舍配置或预定网站),可能需要实现网络爬虫技术来获取和同步数据,这可能涉及HTTP请求、解析HTML内容、存储抓取的数据等内容。
至于具体代码实现,这涉及到编程语言的选择,如Java、Python等。以下是一个简单的Java示例,假设我们使用Spring Boot和JPA进行数据库操作:
```java
// Student实体
@Entity
public class Student {
@Id
private Long id;
private String name;
// 省略getter和setter
}
// 数据访问接口
public interface StudentRepository extends JpaRepository<Student, Long> {
List<Student> findByRoomId(Long roomId);
}
// 后台服务
@RestController
@RequestMapping("/api/students")
public class StudentController {
private final StudentRepository studentRepository;
public StudentController(StudentRepository studentRepository) {
this.studentRepository = studentRepository;
}
@GetMapping("/{roomId}")
public ResponseEntity<List<Student>> getStudentsByRoom(@PathVariable("roomId") Long roomId) {
return new ResponseEntity<>(studentRepository.findByRoomId(roomId), HttpStatus.OK);
}
}
// 如果有爬虫需求,可能需要引入Jsoup库来抓取网页内容
public class WebScraper {
public List<Student> scrapeData(String url) {
// 使用Jsoup或其他库从URL抓取数据
}
}
```
请注意,这只是一个基础框架,实际项目会更复杂,涉及到更多安全性和性能优化的考虑。
阅读全文
相关推荐
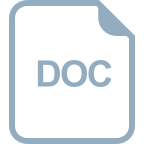
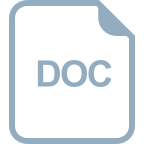
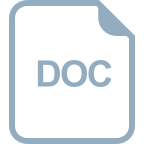

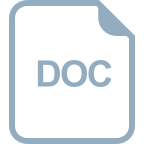
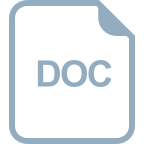
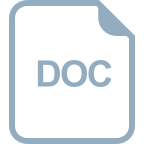
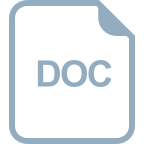
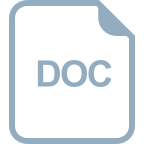
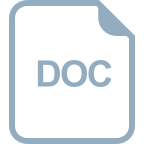
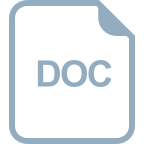
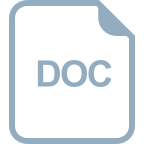
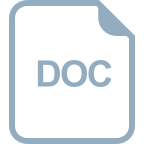
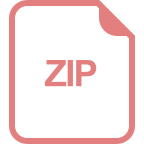
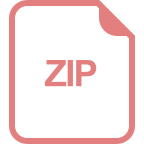
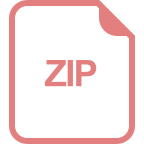
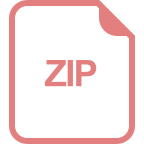
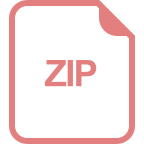