如何在C#中编写一个socket客户端,以实现主动关闭连接的代码?
时间: 2024-10-27 17:08:27 浏览: 43
在C#中,你可以使用`System.Net.Sockets.Socket`类来创建一个Socket客户端并主动关闭连接。下面是一个简单的示例,展示了如何建立连接、发送数据并关闭连接:
```csharp
using System;
using System.Net;
using System.Net.Sockets;
public class SocketClientExample
{
private static readonly IPEndPoint ServerEndPoint = new IPEndPoint(IPAddress.Parse("127.0.0.1"), 12345); // 替换为你服务器的实际IP和端口号
public static void Main()
{
try
{
// 创建一个新的TCP Socket实例
using (Socket client = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp))
{
// 连接到服务器
client.Connect(ServerEndPoint);
Console.WriteLine($"Connected to server at {ServerEndPoint.Address}:{ServerEndPoint.Port}");
// 发送一些数据(这里仅作示例)
byte[] data = Encoding.UTF8.GetBytes("Hello, Server!");
client.Send(data, data.Length, SocketFlags.None);
// 主动关闭连接
client.Shutdown(SocketShutdown.Both);
Console.WriteLine("Closing connection...");
}
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
}
```
在这个例子中,我们首先创建了一个Socket实例,然后使用`Connect`方法连接到指定的服务器地址和端口。接着发送一些数据,最后调用`Shutdown`方法关闭连接的接收和发送功能。
阅读全文
相关推荐
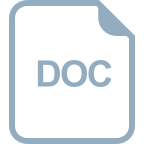
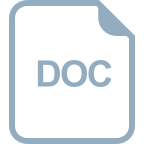
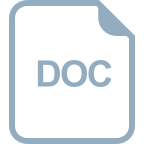
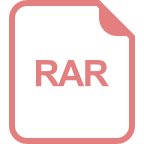
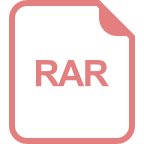
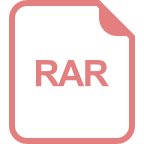
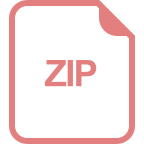
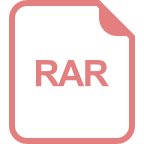
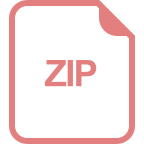
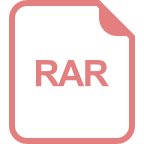
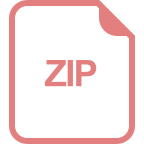
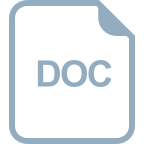
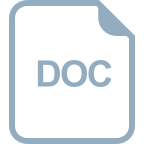





