用数据结构(C语言版)写出按正位序建立一个单链表的算法
时间: 2024-09-23 17:11:13 浏览: 38
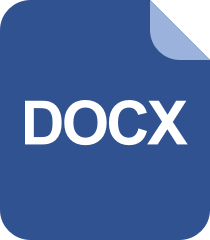
数据结构C语言单链表上实现插入和删除的算法实验报告.docx

在C语言中,我们可以使用循环结构结合链表的基本操作(如创建节点、连接节点)来实现将一个整数数组按照正序构建成单链表的算法。以下是简单的步骤:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 节点值
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建新节点并初始化
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = value;
newNode->next = NULL;
}
return newNode;
}
// 将数组元素插入链表
void buildLinkedList(int arr[], int size, Node** head) {
*head = NULL; // 初始化链表头为NULL
for (int i = 0; i < size; i++) {
Node* newNode = createNode(arr[i]);
if (*head == NULL) { // 如果链表为空,直接插入第一个节点
*head = newNode;
} else { // 非空链表,插入到尾部
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
}
// 打印链表
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
int arr[] = {5, 3, 8, 1, 9};
int size = sizeof(arr) / sizeof(arr[0]);
Node* head = NULL;
buildLinkedList(arr, size, &head);
printList(head);
return 0;
}
```
这个程序首先定义了一个链表节点结构,并实现了创建新节点和构建链表的功能。`buildLinkedList`函数遍历输入数组,将每个元素添加到链表的适当位置。`printList`函数用于查看链表的内容。
阅读全文
相关推荐
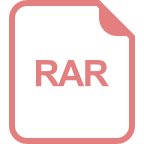
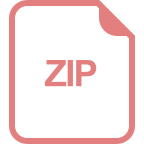
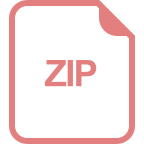
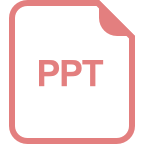
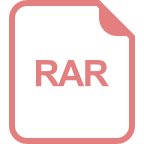
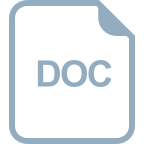
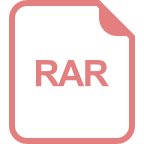
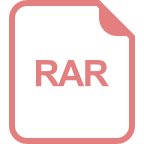
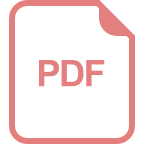
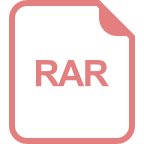
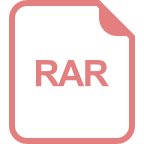
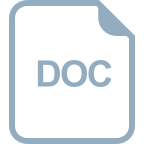
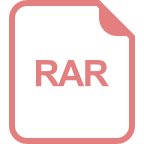
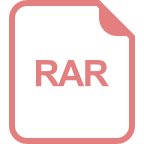
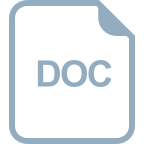
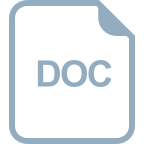
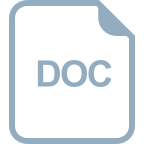