springboot把shp转成geojson
时间: 2024-10-23 12:16:20 浏览: 38
Spring Boot本身不是一个图形处理库,但它可以与第三方库配合,如`spring-cloud-gcp-starter-bigquery`或`jackson-dataformat-cbor`等,来处理数据转换。如果需要将Shapefile (.shp)转换为GeoJSON格式,你可以采用以下步骤:
1. **添加依赖**:首先,你需要在项目中引入支持Shapefile读取和GeoJSON生成的库,比如`geotools`用于处理几何数据,`mapstruct`或者`Jackson`作为数据映射工具。
```xml
<dependency>
<groupId>org.geotools</groupId>
<artifactId>gt-shapefile</artifactId>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-geojson</artifactId>
</dependency>
```
2. **解析Shapefile**:使用Geotools库读取Shapefile内容,获取ShapeRecords(形状记录),每个记录包含几何信息和属性数据。
```java
import org.geotools.data.shapefile.ShapefileDataStore;
import org.locationtech.jts.geom.Geometry;
import java.io.File;
ShapefileDataStore ds = new ShapefileDataStore(new File("path_to_your_shp_file"));
FeatureSource<SimpleFeatureType, SimpleFeature> source = ds.getFeatureSource();
SimpleFeatureCollection features = source.getFeatures();
```
3. **转换为GeoJSON**:遍历features,利用Jackson或其他数据绑定库将每个ShapeRecord转换为GeoJSON Feature对象,然后集合起来形成GeoJSON Feature Collection。
```java
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.type.TypeFactory;
List<Feature> geoJsonFeatures = new ArrayList<>();
for (SimpleFeature feature : features) {
Geometry geometry = (Geometry) feature.getDefaultGeometry();
// 添加属性到Feature对象
PropertyBuilder properties = PropertyBuilder.create();
properties.addAll(feature.getAttributes());
GeoJsonFeature jsonFeature = new GeoJsonFeature(
TypeFactory.defaultInstance().constructType(Feature.class),
"your_feature_id", // 标题
geometry,
properties.buildProperties()
);
geoJsonFeatures.add(jsonFeature);
}
ObjectMapper mapper = new ObjectMapper();
String geoJsonString = mapper.writeValueAsString(new GeoJsonFeatureCollection(features, "your_layer_name"));
```
4. **返回结果**:最后,将生成的GeoJSON字符串返回给前端,或存储到数据库、API响应等地方。
阅读全文
相关推荐
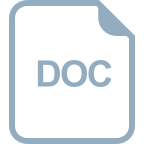
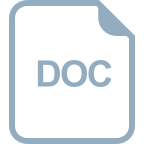
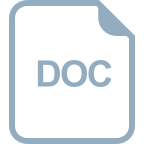
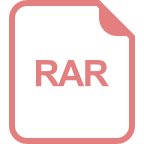
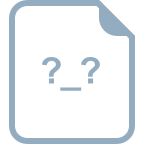
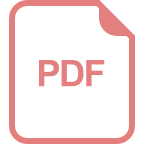
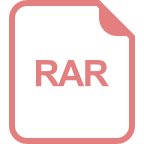
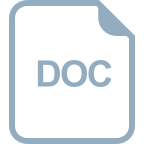
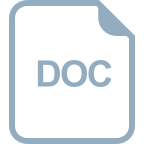
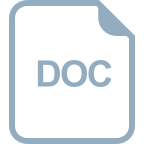
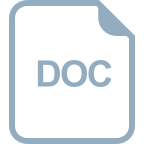
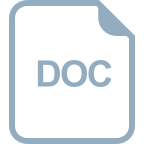




