编写一个Java 函数,实现二分查找算法,在有序数组中查找指定元素,并返回其索 如果未找到,则返回-1
时间: 2024-09-27 11:03:56 浏览: 33
在Java中,你可以编写如下的二分查找函数来实现这一功能:
```java
public class BinarySearchExample {
public static int binarySearch(int[] sortedArray, int target) {
int left = 0; // 左边界
int right = sortedArray.length - 1; // 右边界
while (left <= right) {
int mid = left + (right - left) / 2; // 中间位置
// 如果目标值等于中间值,直接返回索引
if (sortedArray[mid] == target) {
return mid;
}
// 如果目标值小于中间值,更新右边界
else if (sortedArray[mid] > target) {
right = mid - 1;
}
// 否则,目标值大于中间值,更新左边界
else {
left = mid + 1;
}
}
// 没有找到目标值,返回-1
return -1;
}
public static void main(String[] args) {
int[] array = {1, 3, 5, 7, 9}; // 示例的有序数组
int searchValue = 5;
int result = binarySearch(array, searchValue);
if (result != -1) {
System.out.printf("Element found at index %d\n", result);
} else {
System.out.println("Element not found in the array.");
}
}
}
```
在这个例子中,`binarySearch`函数接受一个已排序的整数数组和一个目标值。它通过不断将搜索范围缩小到数组的一半,直到找到目标值或者搜索范围为空(即左右边界相遇),以此来提高查找效率。
阅读全文
相关推荐
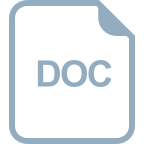
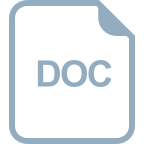
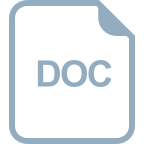


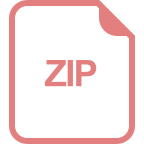
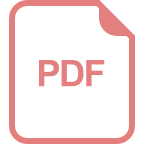
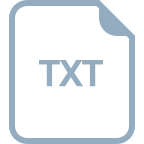
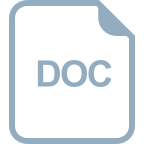
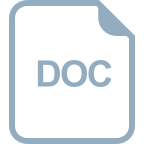
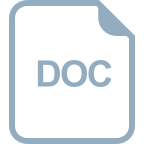
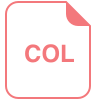
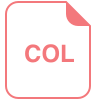
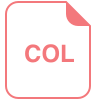
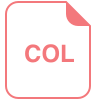
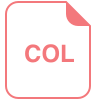


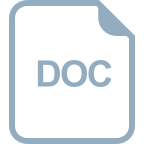
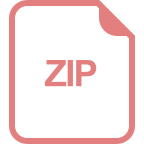