java实现将文件夹压缩
时间: 2023-11-29 16:04:14 浏览: 64
可以使用Java的ZipOutputStream类来实现将文件夹压缩。以下是一个简单的示例代码:
```java
import java.io.*;
import java.util.zip.*;
public class ZipFolder {
public static void main(String[] args) throws Exception {
String sourceFolder = "path/to/source/folder";
String zipFile = "path/to/zip/file.zip";
ZipOutputStream zipOut = new ZipOutputStream(new FileOutputStream(zipFile));
File fileToZip = new File(sourceFolder);
zipFolder(fileToZip, fileToZip.getName(), zipOut);
zipOut.close();
}
private static void zipFolder(File folder, String parentFolder, ZipOutputStream zipOut) throws Exception {
byte[] buffer = new byte[1024];
for (File file : folder.listFiles()) {
if (file.isDirectory()) {
zipFolder(file, parentFolder + "/" + file.getName(), zipOut);
continue;
}
FileInputStream fis = new FileInputStream(file);
zipOut.putNextEntry(new ZipEntry(parentFolder + "/" + file.getName()));
int length;
while ((length = fis.read(buffer)) > 0) {
zipOut.write(buffer, 0, length);
}
zipOut.closeEntry();
fis.close();
}
}
}
```
在这个示例中,我们首先指定要压缩的文件夹路径和压缩后的zip文件路径。然后,我们创建一个ZipOutputStream对象,并将其用于将文件夹压缩成zip文件。然后,我们递归地遍历文件夹中的所有文件和子文件夹,并将它们添加到ZipOutputStream中。最后,我们关闭ZipOutputStream对象。
阅读全文
相关推荐





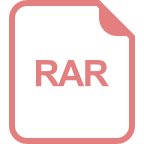






