springboot+vue实现增删改查代码
时间: 2023-07-30 19:10:18 浏览: 66
这是一个比较常见的全栈开发需求,以下是一个简单的示例代码:
后端部分(使用Spring Boot框架):
1. 建立实体类
```java
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@Column(name = "age")
private Integer age;
// getter和setter方法省略
}
```
2. 建立DAO层接口
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
```
3. 建立Service层接口和实现
接口:
```java
public interface UserService {
List<User> findAll();
User findById(Long id);
User save(User user);
void deleteById(Long id);
}
```
实现:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserRepository userRepository;
@Override
public List<User> findAll() {
return userRepository.findAll();
}
@Override
public User findById(Long id) {
return userRepository.findById(id).orElse(null);
}
@Override
public User save(User user) {
return userRepository.save(user);
}
@Override
public void deleteById(Long id) {
userRepository.deleteById(id);
}
}
```
4. 建立Controller层
```java
@RestController
@RequestMapping("/api/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("")
public List<User> findAll() {
return userService.findAll();
}
@GetMapping("/{id}")
public User findById(@PathVariable Long id) {
return userService.findById(id);
}
@PostMapping("")
public User save(@RequestBody User user) {
return userService.save(user);
}
@DeleteMapping("/{id}")
public void deleteById(@PathVariable Long id) {
userService.deleteById(id);
}
}
```
前端部分(使用Vue框架):
1. 建立Vue组件
```vue
<template>
<div>
<div>
<button @click="showAddModal">新增</button>
</div>
<table>
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="user in userList" :key="user.id">
<td>{{ user.id }}</td>
<td>{{ user.name }}</td>
<td>{{ user.age }}</td>
<td>
<button @click="showEditModal(user)">编辑</button>
<button @click="deleteUser(user.id)">删除</button>
</td>
</tr>
</tbody>
</table>
<div v-if="showAdd">
<div>
<label>姓名:</label>
<input v-model="newUser.name">
</div>
<div>
<label>年龄:</label>
<input v-model="newUser.age">
</div>
<div>
<button @click="addUser">确定</button>
<button @click="hideAddModal">取消</button>
</div>
</div>
<div v-if="showEdit">
<div>
<label>姓名:</label>
<input v-model="selectedUser.name">
</div>
<div>
<label>年龄:</label>
<input v-model="selectedUser.age">
</div>
<div>
<button @click="editUser">确定</button>
<button @click="hideEditModal">取消</button>
</div>
</div>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
userList: [],
showAdd: false,
showEdit: false,
newUser: {
name: '',
age: '',
},
selectedUser: null,
};
},
methods: {
showAddModal() {
this.showAdd = true;
},
hideAddModal() {
this.showAdd = false;
this.newUser.name = '';
this.newUser.age = '';
},
addUser() {
axios.post('/api/user', this.newUser)
.then(() => {
this.userList.push(this.newUser);
this.hideAddModal();
})
.catch((err) => {
console.error(err);
});
},
showEditModal(user) {
this.showEdit = true;
this.selectedUser = user;
},
hideEditModal() {
this.showEdit = false;
this.selectedUser = null;
},
editUser() {
axios.put(`/api/user/${this.selectedUser.id}`, this.selectedUser)
.then(() => {
this.hideEditModal();
})
.catch((err) => {
console.error(err);
});
},
deleteUser(id) {
axios.delete(`/api/user/${id}`)
.then(() => {
this.userList = this.userList.filter(user => user.id !== id);
})
.catch((err) => {
console.error(err);
});
},
loadUserList() {
axios.get('/api/user')
.then((res) => {
this.userList = res.data;
})
.catch((err) => {
console.error(err);
});
},
},
mounted() {
this.loadUserList();
},
};
</script>
```
2. 在页面中使用Vue组件
```html
<!DOCTYPE html>
<html>
<head>
<title>用户管理</title>
</head>
<body>
<div id="app">
<user-list></user-list>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<script src="/js/user-list.js"></script>
</body>
</html>
```
以上代码只是一个简单的示例,如果需要更进一步的开发,还需要考虑数据验证、分页、搜索等功能。
相关推荐
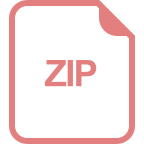
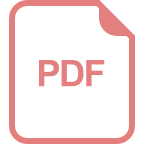














