利用C++求解运输问题
时间: 2023-07-11 08:19:49 浏览: 164
运输问题是一种经典的线性规划问题,可以使用线性规划算法来求解。下面是一个简单的C++代码示例,使用注释进行了解释。
```C++
#include <iostream>
#include <vector>
#include <cmath>
#include <ilcplex/ilocplex.h> // 引入Cplex库
using namespace std;
int main() {
IloEnv env; // 创建Cplex环境
try {
// 定义数据
const int m = 3; // 供应地数量
const int n = 4; // 需求地数量
int s[m] = {100, 150, 200}; // 每个供应地的供应量
int d[n] = {120, 80, 100, 150}; // 每个需求地的需求量
int c[m][n] = {{2, 3, 1, 4}, {1, 4, 2, 3}, {4, 1, 3, 5}}; // 运输成本
// 定义决策变量
IloArray<IloNumVarArray> x(env, m);
for (int i = 0; i < m; i++) {
x[i] = IloNumVarArray(env, n, 0, IloInfinity, ILOFLOAT);
}
// 定义目标函数
IloExpr obj(env);
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
obj += c[i][j] * x[i][j]; // 运输成本乘以运输量
}
}
IloObjective objF(env, obj, IloObjective::Minimize); // 目标函数是最小化运输成本
// 定义约束条件
vector<IloRange> constr; // 用vector存储约束条件
for (int i = 0; i < m; i++) {
IloExpr supply(env);
for (int j = 0; j < n; j++) {
supply += x[i][j]; // 第i个供应地的供应量
}
constr.push_back(IloRange(env, s[i], supply, s[i])); // 约束条件:供应量不能超过供应地的供应量
}
for (int j = 0; j < n; j++) {
IloExpr demand(env);
for (int i = 0; i < m; i++) {
demand += x[i][j]; // 第j个需求地的需求量
}
constr.push_back(IloRange(env, d[j], demand, d[j])); // 约束条件:需求量必须满足需求地的需求量
}
// 定义模型
IloModel model(env);
model.add(objF);
for (int i = 0; i < constr.size(); i++) {
model.add(constr[i]);
}
// 求解模型
IloCplex cplex(model);
cplex.solve();
// 输出结果
cout << "Solution status: " << cplex.getStatus() << endl;
cout << "Objective value: " << cplex.getObjValue() << endl;
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
cout << "x[" << i << "][" << j << "] = " << cplex.getValue(x[i][j]) << endl;
}
}
// 释放内存
obj.end();
for (int i = 0; i < m; i++) {
x[i].end();
}
x.end();
for (int i = 0; i < constr.size(); i++) {
constr[i].end();
}
env.end();
} catch (IloException& e) {
cerr << "Error: " << e.getMessage() << endl;
env.end();
}
return 0;
}
```
这段代码使用了Cplex库来求解运输问题,包括定义决策变量、目标函数和约束条件,以及求解模型并输出结果。需要注意的是,Cplex库需要在系统中安装并配置好,才能正确编译和运行上述代码。
阅读全文
相关推荐
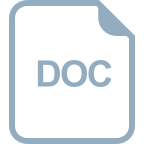
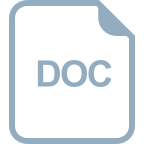
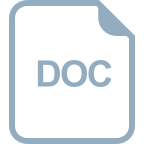

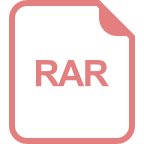
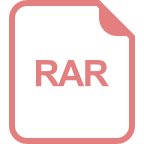
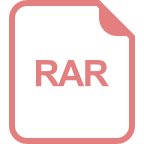
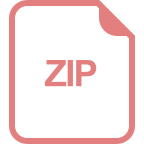
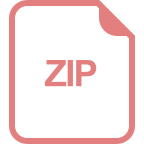
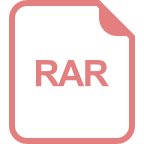
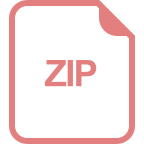
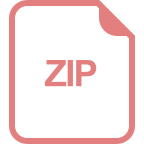
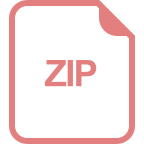
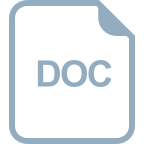
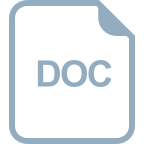
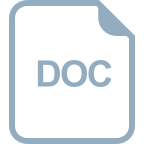
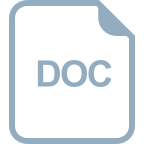
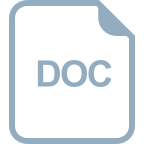
