django-orm-mysql
时间: 2023-10-31 17:29:26 浏览: 45
django-orm-mysql 是一个 Django ORM 扩展,旨在提供更好的 MySQL 数据库支持。它提供了许多 MySQL 特有的功能,如 FULLTEXT 索引支持、JSON 字段支持等。此外,它还提供了一些性能优化,如批量插入和更新,以及使用 InnoDB 引擎的事务支持。如果你在使用 Django 开发 MySQL 数据库应用程序,那么 django-orm-mysql 可能是一个很好的选择。
相关问题
mysql django orm 实例
下面是一个 Django ORM 的示例,使用 MySQL 数据库:
1. 在 settings.py 文件中,指定数据库连接信息:
```python
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'mydatabase',
'USER': 'mydatabaseuser',
'PASSWORD': 'mypassword',
'HOST': 'localhost',
'PORT': '3306',
}
}
```
2. 创建一个模型类,定义数据表的结构和字段:
```python
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.CharField(max_length=50)
publisher = models.CharField(max_length=50)
price = models.DecimalField(max_digits=6, decimal_places=2)
```
3. 使用 Django 的 ORM 进行数据库操作,例如:
```python
# 添加数据
book = Book(title='Python Web 开发', author='Tom', publisher='人民邮电出版社', price=69.9)
book.save()
# 查询数据
books = Book.objects.filter(publisher__contains='邮电')
for book in books:
print(book.title, book.author, book.price)
# 更新数据
book = Book.objects.get(title='Python Web 开发')
book.price = 59.9
book.save()
# 删除数据
book = Book.objects.get(title='Python Web 开发')
book.delete()
```
django-drf搭建Elasticsearch
1. 安装Elasticsearch和Python Elasticsearch客户端
- Elasticsearch安装可以参考官网的[安装文档](https://www.elastic.co/guide/en/elasticsearch/reference/current/install-elasticsearch.html)
- Python Elasticsearch客户端可以使用pip安装:`pip install elasticsearch`
2. 在Django项目中创建一个app,用于处理与Elasticsearch相关的逻辑。
3. 配置Elasticsearch连接信息,可以在Django的`settings.py`中添加以下配置:
```
ELASTICSEARCH_DSL = {
'default': {
'hosts': 'localhost:9200'
},
}
```
其中,`hosts`就是Elasticsearch的地址,这里使用默认的`localhost:9200`。
4. 创建Elasticsearch索引模板,可以在app目录下创建一个`search_indexes.py`文件,定义索引模板:
```
from elasticsearch_dsl import Document, Text, Date, Keyword
class ArticleIndex(Document):
title = Text()
content = Text()
pub_date = Date()
tags = Keyword(multi=True)
class Index:
name = 'articles'
```
其中,`ArticleIndex`是一个继承自`Document`的类,定义了索引的字段和类型。`Index`类中的`name`属性指定了索引的名称。
5. 在app目录下创建`serializers.py`文件,定义序列化器,将模型序列化为Elasticsearch索引模板:
```
from rest_framework import serializers
from .models import Article
from .search_indexes import ArticleIndex
class ArticleIndexSerializer(serializers.ModelSerializer):
class Meta:
model = Article
fields = ('id', 'title', 'content', 'pub_date', 'tags')
def save(self, **kwargs):
article = super().save(**kwargs)
article_index = ArticleIndex(meta={'id': article.id}, **article.__dict__)
article_index.save()
return article
```
其中,`ArticleIndexSerializer`继承自`ModelSerializer`,定义了序列化的模型和字段。在`save`方法中,先保存模型,再将模型数据序列化为Elasticsearch索引模板,最后保存到Elasticsearch中。
6. 在app目录下创建`views.py`文件,定义视图函数,实现Elasticsearch搜索功能:
```
from rest_framework.views import APIView
from rest_framework.response import Response
from elasticsearch_dsl import Q
from .search_indexes import ArticleIndex
from .serializers import ArticleIndexSerializer
class ArticleSearchView(APIView):
def get(self, request):
query = request.query_params.get('q', '')
s = ArticleIndex.search().query(
Q('multi_match', query=query, fields=['title', 'content', 'tags'])
)
response = []
for hit in s.execute().hits:
serializer = ArticleIndexSerializer(data=hit.to_dict())
serializer.is_valid()
response.append(serializer.data)
return Response(response)
```
其中,`ArticleSearchView`继承自`APIView`,定义了一个`get`方法,接收`q`参数作为搜索关键词。通过Elasticsearch DSL构建查询语句,搜索索引模板中的数据,最后将搜索结果序列化返回。
7. 在app目录下创建`urls.py`文件,定义路由:
```
from django.urls import path
from .views import ArticleSearchView
urlpatterns = [
path('search/', ArticleSearchView.as_view(), name='article_search'),
]
```
其中,`search`路由对应了`ArticleSearchView`视图函数。
8. 在Django的`settings.py`中添加app,配置数据库信息:
```
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'rest_framework',
'app_name', # 添加app
]
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'db_name',
'USER': 'db_user',
'PASSWORD': 'db_password',
'HOST': 'localhost',
'PORT': '3306',
}
}
```
9. 在app目录下创建`models.py`文件,定义模型,使用Django ORM作为数据源:
```
from django.db import models
class Article(models.Model):
title = models.CharField(max_length=255)
content = models.TextField()
pub_date = models.DateTimeField(auto_now_add=True)
tags = models.CharField(max_length=255, blank=True)
def __str__(self):
return self.title
```
其中,`Article`是一个继承自`models.Model`的类,定义了文章的属性。
10. 在app目录下创建`admin.py`文件,注册模型到Django Admin中:
```
from django.contrib import admin
from .models import Article
admin.site.register(Article)
```
11. 在Django的`urls.py`中添加app的路由:
```
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('api/', include('app_name.urls')),
]
```
12. 启动Django开发服务器,访问`http://localhost:8000/admin/`,进入Django Admin,创建一些文章数据。
13. 访问`http://localhost:8000/api/search/?q=django`,可以看到搜索结果,其中包含关键词`django`的文章数据。
相关推荐
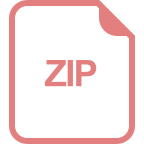
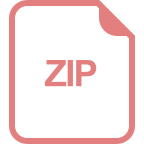












