Implement the function for finding girth of undirected graphs: Given the adjacency lists of an undirected, return the girth if there is any cycle in the graph. Otherwise, return 'inf'. For example: Test Result adj_list = [[1,2],[0,4,5],[0,3],[2,5,6],[1,6],[1,3,6],[3,4,5]] print(find_girth(adj_list)) 3
时间: 2024-03-03 14:48:09 浏览: 62
Sure, here's a Python implementation of the function that finds the girth of an undirected graph given its adjacency list:
```
from collections import deque
def find_girth(adj_list):
n = len(adj_list)
girth = float('inf')
for start in range(n):
visited = [False] * n
dist = [-1] * n
queue = deque([(start, 0)])
visited[start] = True
dist[start] = 0
while queue:
node, d = queue.popleft()
for neighbor in adj_list[node]:
if not visited[neighbor]:
visited[neighbor] = True
dist[neighbor] = d + 1
queue.append((neighbor, d + 1))
elif neighbor != start and dist[neighbor] == d + 1:
girth = min(girth, dist[node] + dist[neighbor] + 1)
return girth if girth < float('inf') else 'inf'
```
The function works by doing a breadth-first search from each node in the graph to find the shortest distance to all other nodes. If during the search it encounters a node that has already been visited, it means there is a cycle in the graph. The girth of the graph is the length of the shortest cycle found. We keep track of the minimum girth seen so far and return it at the end. If no cycles are found, the function returns 'inf'.
阅读全文
相关推荐
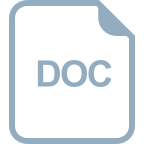
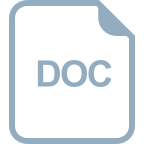
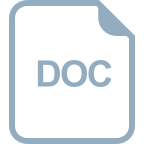

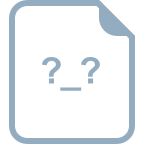
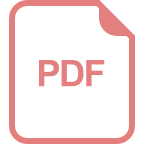
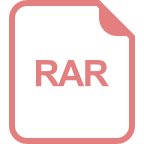
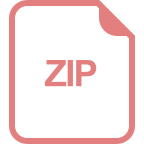
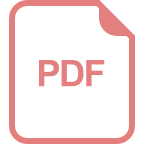
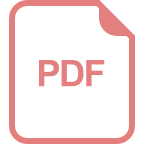
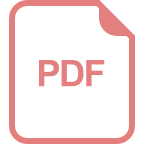
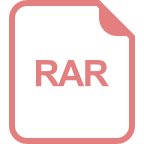
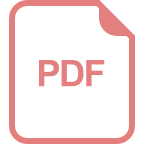
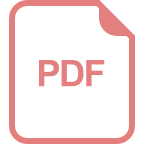
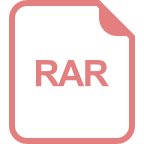
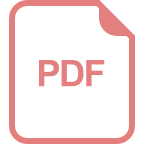
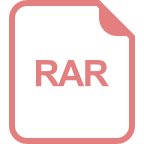
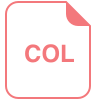
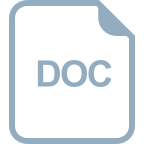