Linux操作系统中利用C++实现多个进程之间互斥访问临界资源(进程个数不限,先写出PV操作的伪算法,然后对这个伪算法进行算法实现)
时间: 2024-03-14 20:45:22 浏览: 18
在Linux操作系统中,可以使用C++语言实现多个进程之间互斥访问临界资源。以下是基于C++的PV操作的实现示例:
```c++
#include <iostream>
#include <cstdlib>
#include <pthread.h>
#include <semaphore.h>
using namespace std;
#define N 5 // 进程个数
#define M 3 // 临界资源个数
sem_t mutex; // 互斥信号量
void* process(void* arg) {
int id = *(int*)arg;
cout << "Process " << id << " is running" << endl;
while (true) {
// 进程申请资源
sem_wait(&mutex);
cout << "Process " << id << " is accessing critical resources" << endl;
// 临界区操作
sleep(1);
cout << "Process " << id << " has released critical resources" << endl;
// 释放资源
sem_post(&mutex);
// 非临界区操作
sleep(1);
}
return NULL;
}
int main() {
pthread_t tid[N];
int ids[N];
sem_init(&mutex, 0, M); // 初始值为M
for (int i = 0; i < N; i++) {
ids[i] = i;
pthread_create(&tid[i], NULL, process, &ids[i]);
}
for (int i = 0; i < N; i++) {
pthread_join(tid[i], NULL);
}
sem_destroy(&mutex);
return 0;
}
```
在上述示例中,使用C++语言实现PV操作,创建N个线程,每个线程通过process函数实现PV操作,实现多个进程之间互斥访问临界资源。其中,互斥信号量mutex的初值为M,表示当前资源可用。当一个线程需要访问临界资源时,先使用sem_wait函数申请资源,如果资源已经被其他线程占用,则当前线程被阻塞,直到其他线程释放资源。当线程完成对临界资源的访问后,使用sem_post函数释放资源,使其他线程可以继续访问。
相关推荐
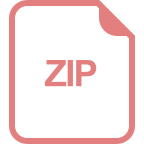














