python操作mongodb数据库
时间: 2023-05-24 21:07:15 浏览: 104
Python中操作MongoDB数据库需要用到pymongo库,安装方法如下:
```
pip install pymongo
```
连接数据库:
```python
from pymongo import MongoClient
# 连接MongoDB数据库
client = MongoClient('mongodb://localhost:27017/')
```
通过client对象获取数据库和集合:
```python
# 获取数据库对象
db = client.testdb
# 获取集合对象(类似于关系数据库中的表)
collection = db.test_collection
```
插入数据:
```python
# 插入一条数据
post = {"author": "Mike",
"text": "My first blog post!",
"tags": ["mongodb", "python", "pymongo"]}
collection.insert_one(post)
# 插入多条数据
new_posts = [{"author": "Mike",
"text": "Another post!",
"tags": ["bulk", "insert"],
"date": datetime.datetime(2009, 11, 12, 11, 14)},
{"author": "Eliot",
"title": "MongoDB is fun",
"text": "and pretty easy too!",
"date": datetime.datetime(2009, 11, 10, 10, 45)}]
collection.insert_many(new_posts)
```
查询数据:
```python
# 查询所有数据
for post in collection.find():
print(post)
# 条件查询
query = {"author": "Mike"}
for post in collection.find(query):
print(post)
# 正则表达式查询
query = {"author": {"$regex": "^M.*"}}
for post in collection.find(query):
print(post)
```
修改数据:
```python
# 更新一条数据
result = collection.update_one(
{"author": "Mike"},
{"$set": {"text": "My first blog post (update)!"}}
)
print("影响的文档数量:", result.modified_count)
# 更新多条数据
result = collection.update_many(
{"author": "Mike"},
{"$set": {"text": "My first blog post (update)!"}}
)
print("影响的文档数量:", result.modified_count)
```
删除数据:
```python
# 删除一条数据
result = collection.delete_one({"author": "Mike"})
print("影响的文档数量:", result.deleted_count)
# 删除多条数据
result = collection.delete_many({"author": "Mike"})
print("影响的文档数量:", result.deleted_count)
# 删除所有数据
result = collection.delete_many({})
print("影响的文档数量:", result.deleted_count)
```
关闭连接:
```python
# 关闭连接
client.close()
```
相关推荐
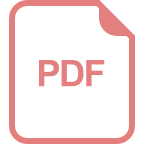
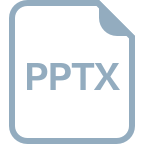
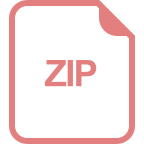














