global img1 img1 = tk.PhotoImage(file="弹幕密度.png") label1 = tk.Label(notebook, image=img1) notebook.add(label1, text="弹幕密度") # 创建一个图片对象,从本地的"弹幕颜色.png"图片中读取数据 global img2 img2 = tk.PhotoImage(file="弹幕颜色.png") # 创建一个标签对象,显示上面创建的图片对象 label2 = tk.Label(notebook, image=img2) # 将标签对象添加到第二个选项卡中 notebook.add(label2, text="弹幕颜色") global img3 img3 = tk.PhotoImage(file="弹幕情感分析.png") label3 = tk.Label(notebook, image=img3) notebook.add(label3, text="弹幕情感分析") global img4 img4 = tk.PhotoImage(file="弹幕词云图.png") label4 = tk.Label(notebook, image=img4) notebook.add(label4, text="弹幕词云图") global ing5 img5 = tk.PhotoImage(file="弹幕句云图.png") label5 = tk.Label(notebook, image=img5) notebook.add(label5, text="弹幕句云图")为什么生成不出选项卡
时间: 2024-04-08 10:33:24 浏览: 38
根据您提供的代码,我注意到在 `img5` 的定义上存在一个拼写错误。您将 `global ing5` 定义为 `img5`。请将其更正为 `global img5`,如下所示:
```python
global img5
img5 = tk.PhotoImage(file="弹幕句云图.png")
label5 = tk.Label(notebook, image=img5)
notebook.add(label5, text="弹幕句云图")
```
此外,请确保您的图片文件 `"弹幕密度.png"`, `"弹幕颜色.png"`, `"弹幕情感分析.png"`, `"弹幕词云图.png"` 和 `"弹幕句云图.png"` 位于正确的路径下,并且文件名的拼写与实际文件名完全一致。这样,您就可以成功地在选项卡中生成图像了。如果问题仍然存在,请提供更多的错误信息,以便我能够帮助您解决问题。
相关问题
import cv2 import tkinter as tk from PIL import Image, ImageTk # 打开摄像头 cap = cv2.VideoCapture(0) # 创建GUI界面 root = tk.Tk() # 定义拍照函数 def take_picture(): ret, frame = cap.read() # 读取摄像头数据 cv2.imwrite("picture.jpg", frame) # 保存图片 img = Image.open("picture.jpg") # 打开图片 img = img.resize((400, 300), Image.ANTIALIAS) # 调整图片大小 photo = ImageTk.PhotoImage(img) # 转换为Tkinter可用的图片格式 label.configure(image=photo) # 更新Label显示的图片 label.image = photo # 创建按钮 button = tk.Button(root, text="拍照", command=take_picture) button.pack() # 创建Label显示摄像头数据 label = tk.Label(root) label.pack() # 循环更新GUI界面 while True: ret, frame = cap.read() # 读取摄像头数据 cv2image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGBA) # 转换颜色格式 img = Image.fromarray(cv2image) # 转换为PIL可用的图片格式 img = img.resize((400, 300), Image.ANTIALIAS) # 调整图片大小 photo = ImageTk.PhotoImage(img) # 转换为Tkinter可用的图片格式 label.configure(image=photo) # 更新Label显示的图片 label.image = photo root.update() # 更新GUI界面 # 释放摄像头资源 cap.release() # 关闭GUI界面 root.mainloop() 改为连续拍照,图片以此命名为1,2,3
您可以通过以下代码实现连续拍照,并以1、2、3等命名图片:
```
import cv2
import tkinter as tk
from PIL import Image, ImageTk
# 打开摄像头
cap = cv2.VideoCapture(0)
# 创建GUI界面
root = tk.Tk()
# 定义拍照函数
def take_picture():
global count
ret, frame = cap.read() # 读取摄像头数据
cv2.imwrite(str(count) + ".jpg", frame) # 保存图片
count += 1
if count > 3:
count = 1
img = Image.open(str(count) + ".jpg") # 打开图片
img = img.resize((400, 300), Image.ANTIALIAS) # 调整图片大小
photo = ImageTk.PhotoImage(img) # 转换为Tkinter可用的图片格式
label.configure(image=photo) # 更新Label显示的图片
label.image = photo
# 创建按钮
button = tk.Button(root, text="拍照", command=take_picture)
button.pack()
# 创建Label显示摄像头数据
label = tk.Label(root)
label.pack()
# 循环更新GUI界面
count = 1
while True:
ret, frame = cap.read() # 读取摄像头数据
cv2image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGBA) # 转换颜色格式
img = Image.fromarray(cv2image) # 转换为PIL可用的图片格式
img = img.resize((400, 300), Image.ANTIALIAS) # 调整图片大小
photo = ImageTk.PhotoImage(img) # 转换为Tkinter可用的图片格式
label.configure(image=photo) # 更新Label显示的图片
label.image = photo
root.update() # 更新GUI界面
# 释放摄像头资源
cap.release()
# 关闭GUI界面
root.mainloop()
```
在该代码中,我们添加了一个全局变量`count`,用于记录当前拍照的序号。在拍照时,我们将图片以序号命名并保存,然后更新`count`的值,以便下一次拍照使用。同时,我们将显示的图片改为从文件中读取,以便实现连续拍照。
代码import cv2 import numpy as np from PIL import Image, ImageTk import tkinter as tk # 创建白色背景 width = 400 height = 400 img = np.zeros((height, width, 3), np.uint8) img.fill(255) # 在白色背景上绘制圆形 center = (200, 200) radius = 100 color = (0, 0, 255) thickness = 2 cv2.circle(img, center, radius, color, thickness) # 将图像从OpenCV格式转换为PIL格式 img_rgb = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) img_pil = Image.fromarray(img_rgb) # 将PIL图像转换为Tkinter图像 img_tk = ImageTk.PhotoImage(img_pil) # 创建Tkinter窗口并在其中显示图像 root = tk.Tk() label = tk.Label(root, image=img_tk) label.pack() root.mainloop(),出现错误:'PhotoImage' object has no attribute '_PhotoImage__photo'
这个错误可能是由于`PhotoImage`对象被垃圾回收器清除了。你可以尝试使用全局变量来保存`PhotoImage`对象,以防止其被垃圾回收。你可以在代码中添加以下两行代码:
```
global img_tk
img_tk = ImageTk.PhotoImage(img_pil)
```
这样就可以解决这个问题了。
阅读全文
相关推荐
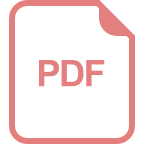
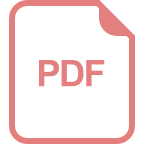
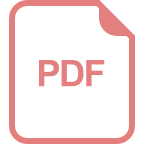
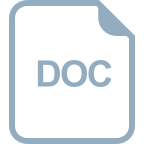
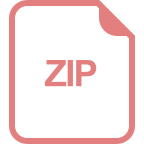
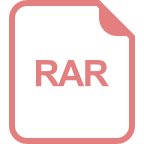
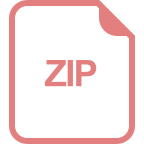
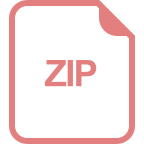
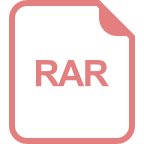
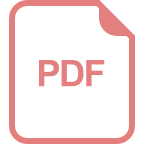
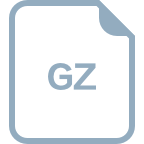
