python运用PIL的直线裁剪算法
时间: 2023-07-25 11:33:15 浏览: 66
使用PIL(Python Imaging Library)的直线裁剪算法可以通过以下步骤实现:
1. 导入PIL库
```python
from PIL import Image, ImageDraw
```
2. 打开图片
```python
im = Image.open("image.png")
```
3. 创建画布和绘图对象
```python
draw = ImageDraw.Draw(im)
```
4. 定义直线的起始点和结束点
```python
x1, y1 = 10, 10
x2, y2 = 100, 100
```
5. 使用绘图对象绘制直线
```python
draw.line((x1, y1, x2, y2), width=5, fill='white')
```
6. 裁剪直线所在的区域
```python
im = im.crop((x1, y1, x2, y2))
```
7. 保存裁剪后的图片
```python
im.save("cropped_image.png")
```
完整示例代码:
```python
from PIL import Image, ImageDraw
im = Image.open("image.png")
draw = ImageDraw.Draw(im)
x1, y1 = 10, 10
x2, y2 = 100, 100
draw.line((x1, y1, x2, y2), width=5, fill='white')
im = im.crop((x1, y1, x2, y2))
im.save("cropped_image.png")
```
相关推荐
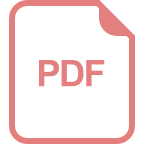
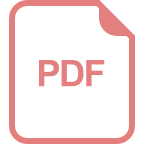
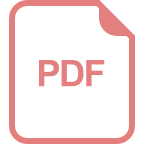














