linux touchpanel驱动demo
时间: 2023-07-25 19:35:05 浏览: 59
以下是一个简单的Linux Touch Panel驱动的demo:
```c
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/init.h>
#include <linux/input.h>
#include <linux/i2c.h>
#include <linux/delay.h>
#define DEVICE_NAME "touchpanel"
static struct i2c_device_id touchpanel_id_table[] = {
{DEVICE_NAME, 0},
{}
};
MODULE_DEVICE_TABLE(i2c, touchpanel_id_table);
static int touchpanel_probe(struct i2c_client *client, const struct i2c_device_id *id)
{
struct input_dev *input;
int error = 0;
input = input_allocate_device();
if (!input) {
dev_err(&client->dev, "input_allocate_device failed\n");
error = -ENOMEM;
goto err_free_mem;
}
input->name = "Touch Panel";
input->id.bustype = BUS_I2C;
input->dev.parent = &client->dev;
input_set_capability(input, EV_KEY, BTN_TOUCH);
error = input_register_device(input);
if (error) {
dev_err(&client->dev, "input_register_device failed\n");
goto err_free_mem;
}
return 0;
err_free_mem:
input_free_device(input);
return error;
}
static int touchpanel_remove(struct i2c_client *client)
{
struct input_dev *input = i2c_get_clientdata(client);
input_unregister_device(input);
input_free_device(input);
return 0;
}
static const struct of_device_id touchpanel_of_match[] = {
{ .compatible = "linux,touchpanel", },
{}
};
MODULE_DEVICE_TABLE(of, touchpanel_of_match);
static struct i2c_driver touchpanel_driver = {
.probe = touchpanel_probe,
.remove = touchpanel_remove,
.id_table = touchpanel_id_table,
.driver = {
.name = DEVICE_NAME,
.of_match_table = touchpanel_of_match,
},
};
module_i2c_driver(touchpanel_driver);
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("Touch Panel Driver");
MODULE_LICENSE("GPL");
```
这个驱动程序使用I2C总线与触摸屏进行通信,并向输入子系统注册输入设备。在`probe`函数中,它分配了一个新的输入设备并配置其名称、总线类型和父设备。它还设置了该设备支持BTN_TOUCH事件。在`remove`函数中,它注销了输入设备并释放了分配的内存。这个驱动程序还包含了一些必要的Linux模块元数据,如作者、描述和许可证信息。
相关推荐
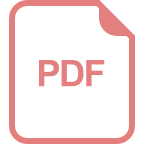











