如何通过Spring Boot将Vue前端图片存入数据库,并再次读取显示?
时间: 2024-03-01 11:54:34 浏览: 29
首先,您需要定义一个包含图片数据的实体类,并使用JPA注解将其映射到数据库中的一张表。例如,以下是一个包含图片数据的实体类的示例:
```java
@Entity
@Table(name = "image")
public class ImageEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@Lob
private byte[] data;
// getters and setters
}
```
其中,`data` 属性使用了 `@Lob` 注解,表示将其映射为数据库中的大对象类型。
接下来,在Spring Boot中定义一个RESTful的API,用于接收前端上传的图片数据,并将其存储到数据库中。例如:
```java
@RestController
@RequestMapping("/api/images")
public class ImageController {
@Autowired
private ImageRepository imageRepository;
@PostMapping("/upload")
public ResponseEntity<?> uploadImage(@RequestParam("file") MultipartFile file) {
try {
ImageEntity imageEntity = new ImageEntity();
imageEntity.setName(file.getOriginalFilename());
imageEntity.setData(file.getBytes());
imageRepository.save(imageEntity);
return ResponseEntity.ok().build();
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("Failed to upload image");
}
}
@GetMapping("/{id}")
public ResponseEntity<byte[]> getImage(@PathVariable("id") Long id) {
Optional<ImageEntity> optional = imageRepository.findById(id);
if (optional.isPresent()) {
ImageEntity imageEntity = optional.get();
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.IMAGE_JPEG);
return new ResponseEntity<>(imageEntity.getData(), headers, HttpStatus.OK);
} else {
return ResponseEntity.notFound().build();
}
}
}
```
其中,`uploadImage` 方法用于接收前端上传的图片数据,并将其存储到数据库中;`getImage` 方法用于根据图片的ID从数据库中读取图片数据,并返回给前端。
最后,在Vue前端中使用 `axios` 或其他HTTP库发送请求,上传图片并显示。例如:
```vue
<template>
<div>
<input type="file" @change="uploadImage">
<img :src="imageUrl" v-if="imageUrl">
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
imageUrl: null
};
},
methods: {
uploadImage(event) {
const file = event.target.files[0];
const formData = new FormData();
formData.append('file', file);
axios.post('/api/images/upload', formData)
.then(() => this.fetchImage())
.catch(error => console.error(error));
},
fetchImage() {
axios.get(`/api/images/${id}`, { responseType: 'arraybuffer' })
.then(response => {
const imageUrl = URL.createObjectURL(new Blob([response.data], { type: 'image/jpeg' }));
this.imageUrl = imageUrl;
})
.catch(error => console.error(error));
}
}
};
</script>
```
其中,`uploadImage` 方法用于上传图片数据;`fetchImage` 方法用于根据图片的ID从后端获取图片数据,并使用 `Blob` 对象将其转换为URL,以便在 `<img>` 标签中显示。
相关推荐
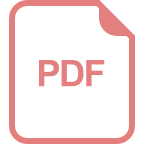
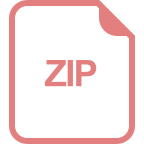
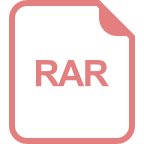














