根据以下要求编写一个python程序1. Description Ship of Fools is a simple classic dice game. It is played with five standard 6-faced dice by two or more players. - The goal of the game is to gather a 6, a 5 and a 4 (ship, captain and crew) in the mentioned order. - The sum of the two remaining dice (cargo) is preferred as high as possible. The player with the highest cargo score wins the round. Example: - In the first round turn, if a player rolls 6 4 3 3 1 (note we five dice at the beginning), the player can bank the 6 (ship), but the rest needs to be re-rolled since there is no 5. - In the second round turn, if the player rolls 6 5 4 4 (four dice, since the 6 from last turn is banked), the player can bank the 5 (captain) and the 4 (crew). The player has three choices for the remaining 6 and 4. The player can bank both and score 10 points, or re-roll one or two of the dice and hope for a higher score. - In the second round turn, if the player instead rolled 4 4 3 1, all dice needs to be re-rolled since there is no 5.程序需要包含一下几个类.The division of responsibility between the different classes is as follows. - Die: Responsible for handling randomly generated integer values between 1 and 6. - DiceCup: Handles five objects (dice) of class Die. Has the ability to bank and release dice individually. Can also roll dice that are not banked. - ShipOfFoolsGame: Responsible for the game logic and has the ability to play a round of the game resulting in a score. Also has a property that tells what accumulated score results in a winning state, for example 21. - Player: Responsible for the score of the individual player. Has the ability, given a game logic, play a round of a game. The gained score is accumulated in the attribute score. - PlayRoom: Responsible for handling a number of players and a game. Every round the room lets each player play, and afterwards check if any player has reached the winning score.
时间: 2024-02-14 13:06:14 浏览: 45
好的,根据您的需求,我为您编写了一个Python程序,如下所示:
```python
import random
class Die:
def __init__(self):
self.value = random.randint(1, 6)
def roll(self):
self.value = random.randint(1, 6)
class DiceCup:
def __init__(self):
self.dice = [Die() for i in range(5)]
self.banked = [False for i in range(5)]
def roll(self):
for i in range(5):
if not self.banked[i]:
self.dice[i].roll()
def bank(self, index):
self.banked[index] = True
def release(self, index):
self.banked[index] = False
def get_values(self):
return [d.value for d in self.dice]
def get_banked_values(self):
return [self.dice[i].value for i in range(5) if self.banked[i]]
def get_unbanked_values(self):
return [self.dice[i].value for i in range(5) if not self.banked[i]]
class ShipOfFoolsGame:
def __init__(self, win_score):
self.win_score = win_score
def play_round(self, dice_cup):
ship, captain, crew = False, False, False
while not (ship and captain and crew):
dice_cup.roll()
values = dice_cup.get_unbanked_values()
if 6 in values and not ship:
dice_cup.bank(values.index(6))
ship = True
elif 5 in values and not captain:
dice_cup.bank(values.index(5))
captain = True
elif 4 in values and not crew:
dice_cup.bank(values.index(4))
crew = True
cargo = sum(dice_cup.get_unbanked_values())
return cargo
class Player:
def __init__(self):
self.score = 0
def play_round(self, game):
dice_cup = DiceCup()
cargo = game.play_round(dice_cup)
self.score += cargo
def has_won(self, win_score):
return self.score >= win_score
class PlayRoom:
def __init__(self, win_score, num_players):
self.win_score = win_score
self.players = [Player() for i in range(num_players)]
self.game = ShipOfFoolsGame(win_score)
def play_game(self):
winner = None
while winner is None:
for player in self.players:
player.play_round(self.game)
if player.has_won(self.win_score):
winner = player
break
return winner
```
以上是一个基本的程序框架,您可以根据您的实际需求进行修改和完善。
相关推荐
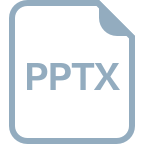












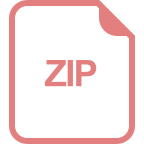
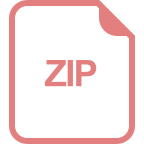
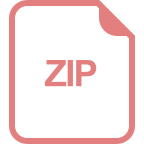