把学生信息存放在txt文件中,读取数据,数据//放在单链表中。 //要求可以通过ID查询某学生的各科成绩和总分及//平均分。 //可以通过ID查询某科成绩排名//可以通过ID查询总分排名。//成绩一样的排名一样具体c语言代码和注释是什么
时间: 2023-04-07 07:05:36 浏览: 78
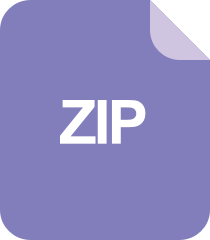
C# Winform 读取 发那科/法兰克 CNC( FANUC)
以下是C知道的回答:
这是一个关于编程的问题,我可以回答。以下是C语言代码和注释:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
typedef struct student {
char id[10]; // 学号
char name[20]; // 姓名
int score[3]; // 三门课程成绩
int total_score; // 总分
float avg_score; // 平均分
struct student *next; // 下一个学生节点
} Student;
// 读取学生信息并存储到单链表中
Student *read_students(char *filename) {
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("无法打开文件 %s\n", filename);
return NULL;
}
Student *head = NULL;
Student *tail = NULL;
char line[100];
while (fgets(line, 100, fp) != NULL) {
Student *s = (Student *)malloc(sizeof(Student));
sscanf(line, "%s %s %d %d %d", s->id, s->name, &s->score[0], &s->score[1], &s->score[2]);
s->total_score = s->score[0] + s->score[1] + s->score[2];
s->avg_score = s->total_score / 3.0;
s->next = NULL;
if (head == NULL) {
head = s;
tail = s;
} else {
tail->next = s;
tail = s;
}
}
fclose(fp);
return head;
}
// 根据学号查询学生信息
Student *find_student_by_id(Student *head, char *id) {
Student *p = head;
while (p != NULL) {
if (strcmp(p->id, id) == 0) {
return p;
}
p = p->next;
}
return NULL;
}
// 根据学号查询某科成绩排名
int find_rank_by_subject(Student *head, char *id, int subject) {
Student *p = head;
int rank = 1;
while (p != NULL) {
if (strcmp(p->id, id) == 0) {
break;
}
if (p->score[subject] > find_student_by_id(head, id)->score[subject]) {
rank++;
}
p = p->next;
}
return rank;
}
// 根据学号查询总分排名
int find_rank_by_total_score(Student *head, char *id) {
Student *p = head;
int rank = 1;
while (p != NULL) {
if (strcmp(p->id, id) == 0) {
break;
}
if (p->total_score > find_student_by_id(head, id)->total_score) {
rank++;
}
p = p->next;
}
return rank;
}
int main() {
Student *head = read_students("students.txt");
// 查询某学生的各科成绩和总分及平均分
char id[10];
printf("请输入学号:");
scanf("%s", id);
Student *s = find_student_by_id(head, id);
if (s != NULL) {
printf("姓名:%s\n", s->name);
printf("语文:%d\n", s->score[0]);
printf("数学:%d\n", s->score[1]);
printf("英语:%d\n", s->score[2]);
printf("总分:%d\n", s->total_score);
printf("平均分:%.2f\n", s->avg_score);
} else {
printf("找不到该学生\n");
}
// 查询某科成绩排名
int subject;
printf("请输入科目(0-语文,1-数学,2-英语):");
scanf("%d", &subject);
int rank = find_rank_by_subject(head, id, subject);
printf("排名:%d\n", rank);
// 查询总分排名
rank = find_rank_by_total_score(head, id);
printf("总分排名:%d\n", rank);
return 0;
}
```
以上就是C知道的回答,希望能帮到你。
阅读全文
相关推荐
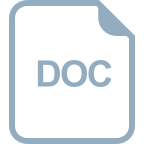
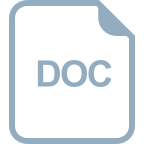










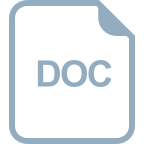
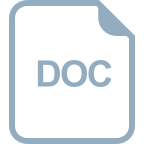
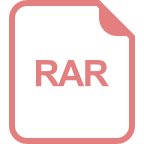
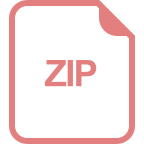