编写一个python程序,爬取豆瓣平台的电影信息,并进行数据分析和可视化界面展示。
时间: 2024-03-07 20:51:27 浏览: 127
好的,下面是一个爬取豆瓣电影信息并进行数据分析和可视化的Python程序:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
import matplotlib.pyplot as plt
# 爬取豆瓣电影TOP250的信息
url = 'https://movie.douban.com/top250'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
# 解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
movies = soup.select('.item')
# 存储电影信息
movie_list = []
for movie in movies:
name = movie.select_one('.title').text.strip()
rating = movie.select_one('.rating_num').text.strip()
director = movie.select_one('.bd p:nth-of-type(1)').text.strip().split(' ')[1]
actors = movie.select_one('.bd p:nth-of-type(1)').text.strip().split(' ')[2:]
actors = '/'.join(actors)
year = movie.select_one('.bd p:nth-of-type(1)').text.strip().split(' ')[-2]
country = movie.select_one('.bd p:nth-of-type(1)').text.strip().split(' ')[-1].replace('(', '').replace(')', '')
summary = movie.select_one('.bd p:nth-of-type(2)').text.strip()
movie_list.append({'name': name, 'rating': rating, 'director': director, 'actors': actors,
'year': year, 'country': country, 'summary': summary})
# 将电影信息存储到CSV文件
movies_df = pd.DataFrame(movie_list)
movies_df.to_csv('movies.csv', index=False)
# 对电影评分进行统计分析和可视化
rating_counts = movies_df['rating'].value_counts()
rating_counts.plot(kind='bar')
plt.title('Douban Movie Rating Counts')
plt.xlabel('Rating')
plt.ylabel('Count')
plt.show()
# 对电影导演进行统计分析和可视化
director_counts = movies_df['director'].value_counts()[:10]
director_counts.plot(kind='bar')
plt.title('Top 10 Movie Directors in Douban TOP250')
plt.xlabel('Director')
plt.ylabel('Count')
plt.show()
```
这个程序使用了Requests库和BeautifulSoup库来爬取豆瓣电影TOP250的信息,并将爬取到的电影信息存储到CSV文件中。接着,使用Pandas库对电影评分和导演进行统计分析,并使用Matplotlib库将统计结果可视化展示出来。
注意:在运行程序之前,需要安装所需要的库,如Requests、BeautifulSoup、Pandas和Matplotlib等。
阅读全文
相关推荐
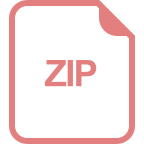
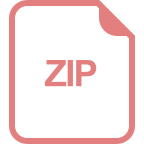
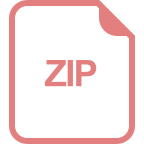















