django+ajax实现进度条的实例
时间: 2023-12-20 08:05:37 浏览: 35
要在Django中实现进度条,可以使用Django框架提供的HttpResponse对象和Ajax技术。
首先,在views.py中编写一个函数,用于处理上传文件的请求。该函数可以使用Python的os模块来执行上传文件的操作,并在上传过程中输出进度信息。
然后,在该函数中使用Django的HttpResponse对象来返回上传进度信息。可以将进度信息转化为JSON格式,并使用HttpResponse对象的content_type参数来指定返回的数据类型。
最后,在前端使用Ajax技术来获取HttpResponse对象中的进度信息,并将其显示在进度条上。
下面是一个简单的示例代码:
```python
# views.py
import os
from django.http import HttpResponse
from django.views.decorators.csrf import csrf_exempt
import json
@csrf_exempt
def upload(request):
if request.method == 'POST':
file = request.FILES.get('file')
file_path = '/path/to/upload/directory/' + file.name
with open(file_path, 'wb+') as f:
for chunk in file.chunks():
f.write(chunk)
uploaded_size = f.tell()
total_size = file.size
progress = uploaded_size / total_size
response_data = {
'progress': progress,
'uploaded_size': uploaded_size,
'total_size': total_size
}
response_json = json.dumps(response_data)
response = HttpResponse(response_json, content_type='application/json')
response['Content-Length'] = len(response_json)
yield response
# upload.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Upload File</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
</head>
<body>
<form id="upload-form" method="POST" enctype="multipart/form-data">
{% csrf_token %}
<input type="file" name="file">
<button type="submit">Upload</button>
</form>
<div>
<progress id="progress-bar" value="0" max="1"></progress>
<span id="progress-text">0%</span>
</div>
<script type="text/javascript">
$(document).ready(function() {
$('#upload-form').submit(function(event) {
event.preventDefault();
var formData = new FormData(this);
$.ajax({
url: '/upload/',
type: 'POST',
data: formData,
processData: false,
contentType: false,
xhr: function() {
var xhr = new window.XMLHttpRequest();
xhr.upload.addEventListener('progress', function(event) {
if (event.lengthComputable) {
var percentComplete = event.loaded / event.total;
$('#progress-bar').val(percentComplete);
$('#progress-text').text(percentComplete * 100 + '%');
}
}, false);
return xhr;
},
success: function(response) {
console.log('Upload complete!');
},
});
});
});
</script>
</body>
</html>
```
在这个例子中,upload函数会处理上传文件的请求,并在上传过程中输出进度信息。在前端,使用Ajax技术来获取HttpResponse对象中的进度信息,并将其显示在进度条上。
通过访问http://yourdomain.com/upload/即可上传文件并动态显示进度条。
相关推荐
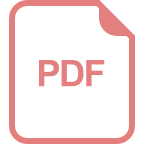
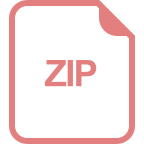
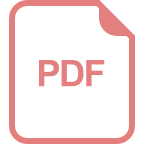
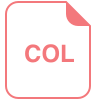
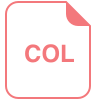
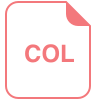











