springboot项目中实现在sql语句的分页(使用RowBounds方式)
时间: 2023-12-16 10:06:02 浏览: 84
在Spring Boot项目中,可以使用MyBatis作为ORM框架来实现在SQL语句的分页。MyBatis提供了RowBounds类来进行分页查询,下面是实现分页查询的步骤:
1. 在MyBatis的mapper文件中编写SQL语句,例如:
```
<select id="getUserList" resultMap="userResultMap">
select * from user
</select>
```
2. 在Spring Boot项目中配置MyBatis,例如:
```
@Configuration
@MapperScan("com.example.demo.mapper")
public class MyBatisConfig {
@Bean
public SqlSessionFactoryBean sqlSessionFactoryBean() throws Exception {
SqlSessionFactoryBean sessionFactory = new SqlSessionFactoryBean();
sessionFactory.setDataSource(dataSource());
sessionFactory.setMapperLocations(new PathMatchingResourcePatternResolver().getResources("classpath*:mapper/*.xml"));
return sessionFactory;
}
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName("com.mysql.jdbc.Driver");
dataSource.setUrl("jdbc:mysql://localhost:3306/test");
dataSource.setUsername("root");
dataSource.setPassword("root");
return dataSource;
}
}
```
3. 在mapper文件中使用RowBounds进行分页查询,例如:
```
<select id="getUserList" resultMap="userResultMap">
select * from user
<where>
<if test="name != null and name != ''">
and name like concat('%', #{name}, '%')
</if>
</where>
order by id desc
<if test="startIndex != null and pageSize != null">
limit #{startIndex}, #{pageSize}
</if>
</select>
```
4. 在Java代码中调用mapper方法进行分页查询,例如:
```
@Autowired
private UserMapper userMapper;
public List<User> getUserList(String name, Integer startIndex, Integer pageSize) {
RowBounds rowBounds = new RowBounds(startIndex, pageSize);
return userMapper.getUserList(name, rowBounds);
}
```
其中,startIndex和pageSize是分页查询的参数,需要在mapper中使用if标签进行判断,如果有值则使用limit关键字进行分页查询。getUserList方法中使用RowBounds对象进行分页查询。
阅读全文
相关推荐
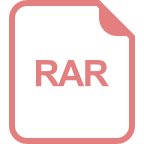
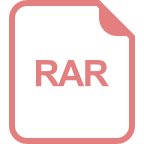


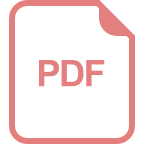
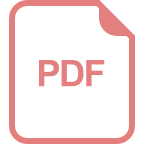
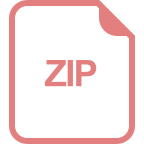
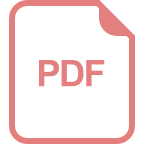
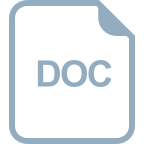
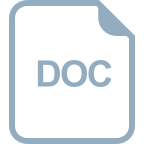
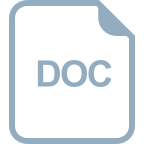







