用pygame写一个跳棋游戏
时间: 2023-11-16 09:04:11 浏览: 52
好的,以下是一个简单的跳棋游戏的代码示例,使用Pygame库编写:
```python
import pygame
# 初始化Pygame
pygame.init()
# 设置游戏屏幕大小
screen_width = 600
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏标题
pygame.display.set_caption("跳棋游戏")
# 定义游戏棋盘
board = [[0, 1, 0, 1, 0, 1, 0, 1],
[1, 0, 1, 0, 1, 0, 1, 0],
[0, 1, 0, 1, 0, 1, 0, 1],
[0, 0, 1, 0, 1, 0, 1, 0],
[0, 1, 0, 0, 0, 1, 0, 1],
[1, 0, 1, 0, 1, 0, 0, 0],
[0, 1, 0, 1, 0, 1, 0, 1],
[1, 0, 1, 0, 1, 0, 1, 0]]
# 定义游戏棋子
piece_size = 50
red_piece = pygame.image.load("red_piece.png")
red_piece = pygame.transform.scale(red_piece, (piece_size, piece_size))
black_piece = pygame.image.load("black_piece.png")
black_piece = pygame.transform.scale(black_piece, (piece_size, piece_size))
# 定义游戏状态
game_over = False
turn = "red"
# 游戏主循环
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
row = y // piece_size
col = x // piece_size
if board[row][col] == 1: # 点击的是棋子
# 判断是否轮到这个棋子
if (turn == "red" and row < 4) or (turn == "black" and row >= 4):
continue
# 判断是否可以移动
if row-2 >= 0 and col-2 >= 0 and board[row-1][col-1] == 1 and board[row-2][col-2] == 0:
board[row][col] = 0
board[row-1][col-1] = 0
board[row-2][col-2] = 1
turn = "black" if turn == "red" else "red"
elif row-2 >= 0 and col+2 <= 7 and board[row-1][col+1] == 1 and board[row-2][col+2] == 0:
board[row][col] = 0
board[row-1][col+1] = 0
board[row-2][col+2] = 1
turn = "black" if turn == "red" else "red"
elif row+2 <= 7 and col-2 >= 0 and board[row+1][col-1] == 1 and board[row+2][col-2] == 0:
board[row][col] = 0
board[row+1][col-1] = 0
board[row+2][col-2] = 1
turn = "black" if turn == "red" else "red"
elif row+2 <= 7 and col+2 <= 7 and board[row+1][col+1] == 1 and board[row+2][col+2] == 0:
board[row][col] = 0
board[row+1][col+1] = 0
board[row+2][col+2] = 1
turn = "black" if turn == "red" else "red"
elif board[row][col] == 0: # 点击的是空白位置
# 判断是否有选中的棋子
for i in range(8):
for j in range(8):
if board[i][j] == 2:
# 判断是否可以移动
if i-row == 2 and j-col == 2 and board[i-1][j-1] == 1 and board[i-2][j-2] == 0:
board[i][j] = 0
board[i-1][j-1] = 0
board[i-2][j-2] = 2
turn = "black" if turn == "red" else "red"
elif i-row == 2 and j-col == -2 and board[i-1][j+1] == 1 and board[i-2][j+2] == 0:
board[i][j] = 0
board[i-1][j+1] = 0
board[i-2][j+2] = 2
turn = "black" if turn == "red" else "red"
elif i-row == -2 and j-col == 2 and board[i+1][j-1] == 1 and board[i+2][j-2] == 0:
board[i][j] = 0
board[i+1][j-1] = 0
board[i+2][j-2] = 2
turn = "black" if turn == "red" else "red"
elif i-row == -2 and j-col == -2 and board[i+1][j+1] == 1 and board[i+2][j+2] == 0:
board[i][j] = 0
board[i+1][j+1] = 0
board[i+2][j+2] = 2
turn = "black" if turn == "red" else "red"
# 判断是否有选中的棋子
if board[row][col] == 1:
for i in range(8):
for j in range(8):
if board[i][j] == 2:
board[i][j] = 1
board[row][col] = 2
# 绘制游戏界面
screen.fill((255, 255, 255))
for i in range(8):
for j in range(8):
if board[i][j] == 1:
screen.blit(red_piece, (j*piece_size, i*piece_size))
elif board[i][j] == 2:
pygame.draw.rect(screen, (0, 0, 255), (j*piece_size, i*piece_size, piece_size, piece_size), 2)
screen.blit(red_piece if turn == "red" else black_piece, (j*piece_size, i*piece_size))
pygame.display.update()
# 退出Pygame
pygame.quit()
```
注意,这里的代码只是一个简单的示例,并没有完全实现跳棋游戏的所有规则和功能。如果需要实现更完善的跳棋游戏,还需要进行更多的开发和测试。
相关推荐
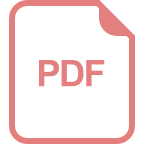














