将译码规律修改为:将一个字母被它前面第 4 个字母代替,例如'E'用'A'代替,Z用'U'代替,'D'用'Z'代替,'C'用'Y'代替,'B'用'X'代替,'A'用'V'代替。修改程序并运行。
时间: 2024-10-29 17:23:41 浏览: 29
要实现这个译码规则,你需要遍历字符串中的每个字符,找到其在字母表中的位置(如果字符不是小写字母,先转换为小写),然后根据给定的替换规则进行计算新的位置。对于字母表中的前四个字母,我们需要特殊处理,因为它们的替换规则不同。
以下是一个简单的C++程序示例,用于完成这个翻译过程:
```cpp
#include <iostream>
#include <string>
std::string decode(const std::string& input) {
std::string output = "";
for (char c : input) {
if ('A' <= c && c <= 'Z') { // 处理大写字母
if (c == 'E')
output += 'A';
else if (c == 'Z')
output += 'U';
else if (c == 'D')
output += 'Z';
else if (c == 'C')
output += 'Y';
else if (c == 'B')
output += 'X';
else if (c == 'A')
output += 'V';
else
output += static_cast<char>((c - 'A' + 4) % 26 + 'A'); // 前四个字母处理后剩余的
} else if ('a' <= c && c <= 'z') { // 处理小写字母
if (c == 'e')
output += 'a';
else if (c == 'z')
output += 'u';
else if (c == 'd')
output += 'z';
else if (c == 'c')
output += 'y';
else if (c == 'b')
output += 'x';
else if (c == 'a')
output += 'v';
else
output += static_cast<char>((c - 'a' + 4) % 26 + 'a');
} else {
output += c; // 非字母字符保持不变
}
}
return output;
}
int main() {
std::string input = "Hello, World!";
std::string decoded = decode(input);
std::cout << "Decoded message: " << decoded << std::endl;
return 0;
}
```
在这个程序中,`decode` 函数接受一个字符串参数,逐个处理其中的字符,按照指定的替换规则进行编码。`main` 函数则演示了如何使用这个函数解码一个例子字符串。
阅读全文
相关推荐
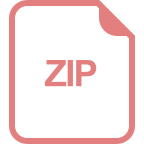
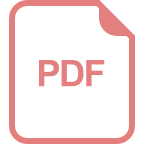
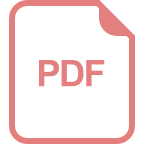









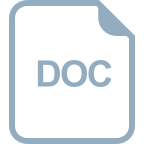
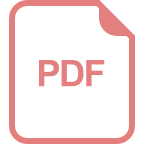
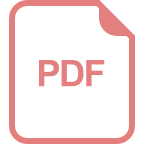
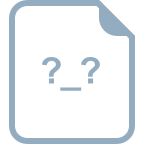