单项选择题标准化考试c语言编程
时间: 2023-09-18 08:15:54 浏览: 99
好的,以下是一个简单的单项选择题标准化考试系统的 C 语言编程示例,它可以随机生成题目、计算得分和统计考试结果:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define NUM_QUESTIONS 10 // 题目数量
#define NUM_OPTIONS 4 // 选项数量
// 题目结构体
typedef struct {
char question[100]; // 题目内容
char options[NUM_OPTIONS][50]; // 选项内容
int answer; // 正确答案
} Question;
// 随机生成题目
void generate_questions(Question *questions) {
int i, j, k;
char *options = "ABCD";
srand(time(NULL)); // 以当前时间为随机数种子
for (i = 0; i < NUM_QUESTIONS; i++) {
// 生成题目内容
sprintf(questions[i].question, "第%d题:以下哪个是正确的?", i + 1);
// 生成选项
for (j = 0; j < NUM_OPTIONS; j++) {
sprintf(questions[i].options[j], "%c. 选项%d", options[j], j + 1);
}
// 随机生成答案
questions[i].answer = rand() % NUM_OPTIONS;
// 将正确答案的选项打乱顺序
k = rand() % NUM_OPTIONS;
if (k != questions[i].answer) {
strcpy(questions[i].options[k], questions[i].options[questions[i].answer]);
strcpy(questions[i].options[questions[i].answer], questions[i].options[k]);
questions[i].answer = k;
}
}
}
// 显示题目
void show_question(Question question) {
int i;
printf("%s\n", question.question);
for (i = 0; i < NUM_OPTIONS; i++) {
printf("%s\n", question.options[i]);
}
}
// 检查答案是否正确
int check_answer(Question question, int answer) {
return answer == question.answer;
}
int main() {
Question questions[NUM_QUESTIONS];
int i, answer, score = 0;
// 随机生成题目
generate_questions(questions);
// 显示题目并记录得分
for (i = 0; i < NUM_QUESTIONS; i++) {
printf("第%d题:\n", i + 1);
show_question(questions[i]);
printf("请输入答案(A/B/C/D):");
scanf("%d", &answer);
if (check_answer(questions[i], answer - 1)) {
printf("回答正确!\n");
score++;
} else {
printf("回答错误!\n");
}
}
// 输出得分和统计结果
printf("您的得分是:%d/%d\n", score, NUM_QUESTIONS);
if (score >= 9) {
printf("您的成绩为优秀!\n");
} else if (score >= 7) {
printf("您的成绩为良好!\n");
} else if (score >= 5) {
printf("您的成绩为及格!\n");
} else {
printf("您的成绩为不及格!\n");
}
return 0;
}
```
你可以将上述代码复制到 C 语言编译器中运行,即可体验这个简单的单项选择题标准化考试系统。
阅读全文
相关推荐
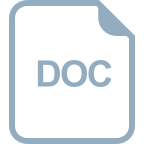
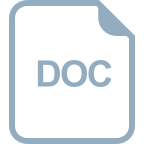
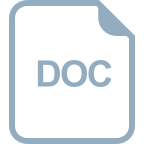
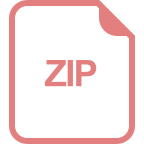
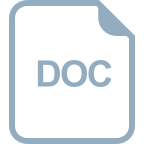

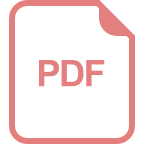
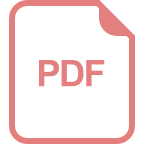
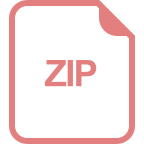
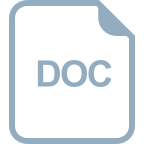
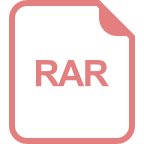
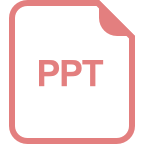
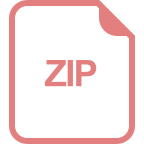
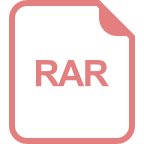
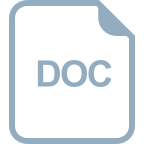
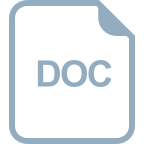
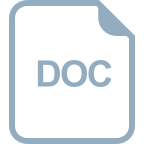
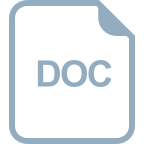