springboot框架实现定时任务有几种方式
时间: 2023-07-12 09:02:56 浏览: 72
Spring Boot框架实现定时任务有三种方式:
1. 使用@Scheduled注解:可以在方法上添加@Scheduled注解,设置定时任务的执行时间和频率。
2. 实现Runnable接口:创建一个实现Runnable接口的类,在run方法中实现定时任务的逻辑,使用Java的ScheduledExecutorService定时执行任务。
3. 实现SchedulingConfigurer接口:实现SchedulingConfigurer接口的configureTasks方法,使用TaskScheduler配置定时任务。
相关问题
基于springboot开发实现定时任务的几种方式 具体代码写出来
基于Spring Boot开发实现定时任务的几种方式有:@Scheduled注解、使用Cron表式、使用Quartz框架。
1. 使用@Scheduled注解:
```java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class ScheduledTasks {
@Scheduled(fixedRate = 1000) // 每隔1秒执行一次
public void task1() {
// 执行任务内容
}
@Scheduled(cron = "0 0 12 * * ?") // 每天中午12点执行
public void task2() {
// 执行任务内容
}
}
```
2. 使用Cron表达式:
```java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class ScheduledTasks {
@Scheduled(cron = "0/5 * * * * ?") // 每隔5秒执行一次
public void task1() {
// 执行任务内容
}
@Scheduled(cron = "0 0 12 * * ?") // 每天中午12点执行
public void task2() {
// 执行任务内容
}
}
```
3. 使用Quartz框架:
首先,需要添加Quartz的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-quartz</artifactId>
</dependency>
```
然后,创建定时任务类:
```java
import org.quartz.Job;
import org.quartz.JobExecutionContext;
import org.quartz.JobExecutionException;
import org.springframework.scheduling.quartz.QuartzJobBean;
public class MyJob extends QuartzJobBean {
@Override
protected void executeInternal(JobExecutionContext jobExecutionContext) throws JobExecutionException {
// 执行任务内容
}
}
```
最后,在配置类中配置定时任务:
```java
import org.quartz.JobDetail;
import org.quartz.SimpleTrigger;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.scheduling.quartz.JobDetailFactoryBean;
import org.springframework.scheduling.quartz.SimpleTriggerFactoryBean;
@Configuration
public class QuartzConfig {
@Bean
public JobDetailFactoryBean jobDetail() {
JobDetailFactoryBean factory = new JobDetailFactoryBean();
factory.setJobClass(MyJob.class);
factory.setDurability(true);
return factory;
}
@Bean
public SimpleTriggerFactoryBean trigger(JobDetail job) {
SimpleTriggerFactoryBean factory = new SimpleTriggerFactoryBean();
factory.setJobDetail(job);
factory.setRepeatInterval(5000); // 每隔5秒执行一次
return factory;
}
}
```
以上就是基于Spring Boot开发实现定时任务的几种方式的代码示例。
springboot 开启定时任务的几种方式
Spring Boot提供了多种方式来开启定时任务,主要包括以下几种:
1. 使用@EnableScheduling注解和@Scheduled注解
@EnableScheduling注解用于开启Spring定时任务的支持,@Scheduled注解则用于指定定时任务的执行时间。这种方式相对简单,适用于定时任务比较简单的场景。
2. 使用TaskScheduler和Runnable接口
TaskScheduler是Spring提供的任务调度器接口,通过实现该接口并使用Runnable接口来定义定时任务的执行逻辑。这种方式比较灵活,适用于定时任务比较复杂的场景。
3. 使用Cron表达式
使用Cron表达式可以更加精确地定义定时任务的执行时间,同时也可以实现定时任务的复杂逻辑。Spring Boot提供了Cron表达式的支持,可以通过@Configuration注解和@Scheduled注解来实现。
4. 使用Quartz框架
Quartz是一个功能强大的定时任务框架,可以满足各种复杂的定时任务需求。Spring Boot提供了Quartz的支持,可以通过添加依赖和配置文件来快速集成Quartz框架。
相关推荐
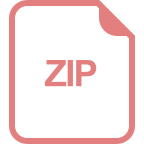
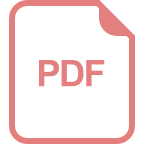
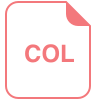
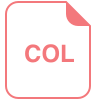










