c语言代码哲学家就餐用进程实现
时间: 2023-10-08 18:08:02 浏览: 105
这是一个经典的哲学家就餐问题,可以使用进程来模拟解决。下面是一个使用C语言实现的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
#include <time.h>
#define N 5
#define LEFT (i+N-1)%N
#define RIGHT (i+1)%N
#define THINKING 0
#define HUNGRY 1
#define EATING 2
int state[N];
pid_t philosophers[N];
void test(int i)
{
if (state[i] == HUNGRY && state[LEFT] != EATING && state[RIGHT] != EATING)
{
state[i] = EATING;
printf("Philosopher %d is eating\n", i);
}
}
void take_forks(int i)
{
state[i] = HUNGRY;
printf("Philosopher %d is hungry\n", i);
test(i);
}
void put_forks(int i)
{
state[i] = THINKING;
printf("Philosopher %d is thinking\n", i);
test(LEFT);
test(RIGHT);
}
void philosopher(int i)
{
while (1)
{
printf("Philosopher %d is thinking\n", i);
sleep(rand() % 5 + 1);
take_forks(i);
sleep(rand() % 5 + 1);
put_forks(i);
}
}
int main()
{
srand(time(NULL));
int i;
for (i = 0; i < N; i++)
{
philosophers[i] = fork();
if (philosophers[i] == 0)
{
philosopher(i);
}
}
for (i = 0; i < N; i++)
{
waitpid(philosophers[i], NULL, 0);
}
return 0;
}
```
这个程序中,首先定义了5个哲学家和他们的状态。每个哲学家都是一个进程,使用 `fork()` 创建。每个哲学家在一个循环中思考、拿起筷子、吃饭、放下筷子,直到进程结束。`test()` 函数用来检查是否可以进餐,如果可以,则设置状态为 EATING ,并输出相应信息。`take_forks()` 和 `put_forks()` 函数分别用来拿起和放下筷子,并设置相应的状态。
需要注意的是,在这个程序中,每个哲学家都是一个进程,它们之间通过共享数据来进行通信。因此,在不同的操作之间需要加锁来保护共享数据。这里没有实现锁机制,是因为这个例子只是为了演示哲学家就餐问题的解决方案,实际应用中需要加上锁来保证进程间的同步和互斥。
阅读全文
相关推荐
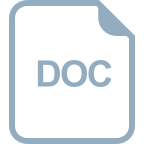
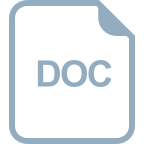
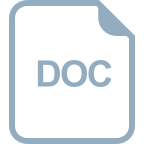
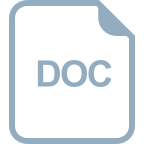
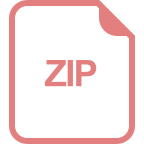
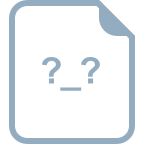
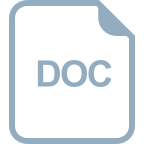
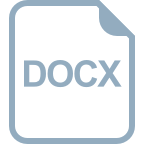
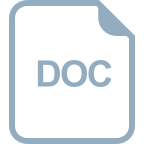
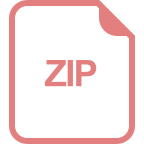
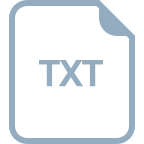
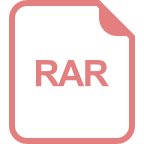
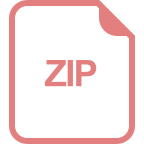