uniapp 使用Vue的 computed
时间: 2023-08-06 09:04:14 浏览: 113
Uniapp 是基于 Vue 的框架,因此可以使用 Vue 的 computed 属性进行计算属性的定义。在 Uniapp 中,我们可以在页面的 script 标签中使用 computed 属性来定义计算属性,例如:
```javascript
export default {
data() {
return {
num1: 10,
num2: 20
}
},
computed: {
sum() {
return this.num1 + this.num2
}
}
}
```
在上面的代码中,我们定义了两个变量 num1 和 num2,并使用 computed 属性定义了一个计算属性 sum,它返回 num1 和 num2 的和。在页面中,我们可以直接使用 sum 变量来获取计算结果。
```html
<template>
<div>{{ sum }}</div>
</template>
```
这样就可以在页面中渲染出计算结果了。需要注意的是,在计算属性中,我们只需要定义计算逻辑,而不需要关心数据的更新,Vue 会自动帮我们处理数据的更新。
相关问题
uniapp使用vue3
作为一个AI语言模型,我不知道uniapp是否支持Vue3,但是根据官方文档,Uni App目前支持 Vue 2.x 版本,未来可能会支持 Vue 3.x 版本。
如果想在Uni App中使用Vue 3,可以尝试手动安装Vue 3并配置项目。需要注意的是,Vue 3与Vue 2在语法上有一些区别,需要进行适当的修改。
以下是一个简单的示例,展示了如何在Uni App中使用Vue 3:
1. 安装Vue 3
```
npm install vue@next
```
2. 在main.js中导入Vue 3
```
import { createApp } from 'vue'
import App from './App.vue'
const app = createApp(App)
app.mount('#app')
```
3. 在组件中使用Vue 3
```
<template>
<div>
{{ message }}
</div>
</template>
<script>
import { reactive } from 'vue'
export default {
setup() {
const state = reactive({
message: 'Hello Vue 3!'
})
return {
...state
}
}
}
</script>
```
需要注意的是,在Vue 3中,组件选项中的data、methods、computed等属性都被移除,需要使用setup函数来实现组件的逻辑。同时,Vue 3也提供了一些新的API,比如ref、reactive、computed等,可以在setup函数中使用。
uniapp的vue2怎么监听数据执行时间
你可以使用Vue.js提供的watch或computed属性来监听数据的变化,计算执行时间。
使用watch:
```
<template>
<div>
<p>{{ myData }}</p>
<p>{{ timeTaken }}ms</p>
</div>
</template>
<script>
export default {
data() {
return {
myData: 'Hello World',
startTime: 0,
endTime: 0,
timeTaken: 0
};
},
watch: {
myData(newValue, oldValue) {
this.startTime = new Date().getTime();
// Do something with newValue or oldValue
this.endTime = new Date().getTime();
this.timeTaken = this.endTime - this.startTime;
}
}
};
</script>
```
使用computed:
```
<template>
<div>
<p>{{ myData }}</p>
<p>{{ timeTaken }}ms</p>
</div>
</template>
<script>
export default {
data() {
return {
myData: 'Hello World',
startTime: 0,
endTime: 0
};
},
computed: {
timeTaken() {
this.startTime = new Date().getTime();
// Do something with this.myData
this.endTime = new Date().getTime();
return this.endTime - this.startTime;
}
}
};
</script>
```
以上两种方式都可以监听数据的变化并计算执行时间,具体使用哪种方式取决于你的需求。
阅读全文
相关推荐
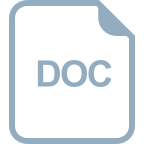
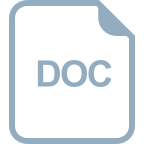
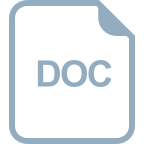
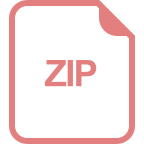
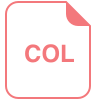











